mirror of
https://github.com/gradle/gradle-build-action.git
synced 2024-10-06 11:46:48 +00:00
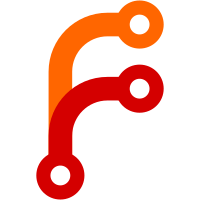
in a best effort manner by default allowing to specify files to hash for computing the cache key
1 line
No EOL
106 KiB
JavaScript
1 line
No EOL
106 KiB
JavaScript
module.exports=function(e,t){"use strict";var r={};function __webpack_require__(t){if(r[t]){return r[t].exports}var n=r[t]={i:t,l:false,exports:{}};var i=true;try{e[t].call(n.exports,n,n.exports,__webpack_require__);i=false}finally{if(i)delete r[t]}n.l=true;return n.exports}__webpack_require__.ab=__dirname+"/";function startup(){return __webpack_require__(878)}return startup()}({1:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};Object.defineProperty(t,"__esModule",{value:true});const i=r(129);const o=r(622);const s=r(669);const a=r(672);const c=s.promisify(i.exec);function cp(e,t,r={}){return n(this,void 0,void 0,function*(){const{force:n,recursive:i}=readCopyOptions(r);const s=(yield a.exists(t))?yield a.stat(t):null;if(s&&s.isFile()&&!n){return}const c=s&&s.isDirectory()?o.join(t,o.basename(e)):t;if(!(yield a.exists(e))){throw new Error(`no such file or directory: ${e}`)}const u=yield a.stat(e);if(u.isDirectory()){if(!i){throw new Error(`Failed to copy. ${e} is a directory, but tried to copy without recursive flag.`)}else{yield cpDirRecursive(e,c,0,n)}}else{if(o.relative(e,c)===""){throw new Error(`'${c}' and '${e}' are the same file`)}yield copyFile(e,c,n)}})}t.cp=cp;function mv(e,t,r={}){return n(this,void 0,void 0,function*(){if(yield a.exists(t)){let n=true;if(yield a.isDirectory(t)){t=o.join(t,o.basename(e));n=yield a.exists(t)}if(n){if(r.force==null||r.force){yield rmRF(t)}else{throw new Error("Destination already exists")}}}yield mkdirP(o.dirname(t));yield a.rename(e,t)})}t.mv=mv;function rmRF(e){return n(this,void 0,void 0,function*(){if(a.IS_WINDOWS){try{if(yield a.isDirectory(e,true)){yield c(`rd /s /q "${e}"`)}else{yield c(`del /f /a "${e}"`)}}catch(e){if(e.code!=="ENOENT")throw e}try{yield a.unlink(e)}catch(e){if(e.code!=="ENOENT")throw e}}else{let t=false;try{t=yield a.isDirectory(e)}catch(e){if(e.code!=="ENOENT")throw e;return}if(t){yield c(`rm -rf "${e}"`)}else{yield a.unlink(e)}}})}t.rmRF=rmRF;function mkdirP(e){return n(this,void 0,void 0,function*(){yield a.mkdirP(e)})}t.mkdirP=mkdirP;function which(e,t){return n(this,void 0,void 0,function*(){if(!e){throw new Error("parameter 'tool' is required")}if(t){const t=yield which(e,false);if(!t){if(a.IS_WINDOWS){throw new Error(`Unable to locate executable file: ${e}. Please verify either the file path exists or the file can be found within a directory specified by the PATH environment variable. Also verify the file has a valid extension for an executable file.`)}else{throw new Error(`Unable to locate executable file: ${e}. Please verify either the file path exists or the file can be found within a directory specified by the PATH environment variable. Also check the file mode to verify the file is executable.`)}}}try{const t=[];if(a.IS_WINDOWS&&process.env.PATHEXT){for(const e of process.env.PATHEXT.split(o.delimiter)){if(e){t.push(e)}}}if(a.isRooted(e)){const r=yield a.tryGetExecutablePath(e,t);if(r){return r}return""}if(e.includes("/")||a.IS_WINDOWS&&e.includes("\\")){return""}const r=[];if(process.env.PATH){for(const e of process.env.PATH.split(o.delimiter)){if(e){r.push(e)}}}for(const n of r){const r=yield a.tryGetExecutablePath(n+o.sep+e,t);if(r){return r}}return""}catch(e){throw new Error(`which failed with message ${e.message}`)}})}t.which=which;function readCopyOptions(e){const t=e.force==null?true:e.force;const r=Boolean(e.recursive);return{force:t,recursive:r}}function cpDirRecursive(e,t,r,i){return n(this,void 0,void 0,function*(){if(r>=255)return;r++;yield mkdirP(t);const n=yield a.readdir(e);for(const o of n){const n=`${e}/${o}`;const s=`${t}/${o}`;const c=yield a.lstat(n);if(c.isDirectory()){yield cpDirRecursive(n,s,r,i)}else{yield copyFile(n,s,i)}}yield a.chmod(t,(yield a.stat(e)).mode)})}function copyFile(e,t,r){return n(this,void 0,void 0,function*(){if((yield a.lstat(e)).isSymbolicLink()){try{yield a.lstat(t);yield a.unlink(t)}catch(e){if(e.code==="EPERM"){yield a.chmod(t,"0666");yield a.unlink(t)}}const r=yield a.readlink(e);yield a.symlink(r,t,a.IS_WINDOWS?"junction":null)}else if(!(yield a.exists(t))||r){yield a.copyFile(e,t)}})}},9:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=i(r(87));const s=i(r(614));const a=i(r(129));const c=i(r(622));const u=i(r(1));const l=i(r(672));const f=process.platform==="win32";class ToolRunner extends s.EventEmitter{constructor(e,t,r){super();if(!e){throw new Error("Parameter 'toolPath' cannot be null or empty.")}this.toolPath=e;this.args=t||[];this.options=r||{}}_debug(e){if(this.options.listeners&&this.options.listeners.debug){this.options.listeners.debug(e)}}_getCommandString(e,t){const r=this._getSpawnFileName();const n=this._getSpawnArgs(e);let i=t?"":"[command]";if(f){if(this._isCmdFile()){i+=r;for(const e of n){i+=` ${e}`}}else if(e.windowsVerbatimArguments){i+=`"${r}"`;for(const e of n){i+=` ${e}`}}else{i+=this._windowsQuoteCmdArg(r);for(const e of n){i+=` ${this._windowsQuoteCmdArg(e)}`}}}else{i+=r;for(const e of n){i+=` ${e}`}}return i}_processLineBuffer(e,t,r){try{let n=t+e.toString();let i=n.indexOf(o.EOL);while(i>-1){const e=n.substring(0,i);r(e);n=n.substring(i+o.EOL.length);i=n.indexOf(o.EOL)}t=n}catch(e){this._debug(`error processing line. Failed with error ${e}`)}}_getSpawnFileName(){if(f){if(this._isCmdFile()){return process.env["COMSPEC"]||"cmd.exe"}}return this.toolPath}_getSpawnArgs(e){if(f){if(this._isCmdFile()){let t=`/D /S /C "${this._windowsQuoteCmdArg(this.toolPath)}`;for(const r of this.args){t+=" ";t+=e.windowsVerbatimArguments?r:this._windowsQuoteCmdArg(r)}t+='"';return[t]}}return this.args}_endsWith(e,t){return e.endsWith(t)}_isCmdFile(){const e=this.toolPath.toUpperCase();return this._endsWith(e,".CMD")||this._endsWith(e,".BAT")}_windowsQuoteCmdArg(e){if(!this._isCmdFile()){return this._uvQuoteCmdArg(e)}if(!e){return'""'}const t=[" ","\t","&","(",")","[","]","{","}","^","=",";","!","'","+",",","`","~","|","<",">",'"'];let r=false;for(const n of e){if(t.some(e=>e===n)){r=true;break}}if(!r){return e}let n='"';let i=true;for(let t=e.length;t>0;t--){n+=e[t-1];if(i&&e[t-1]==="\\"){n+="\\"}else if(e[t-1]==='"'){i=true;n+='"'}else{i=false}}n+='"';return n.split("").reverse().join("")}_uvQuoteCmdArg(e){if(!e){return'""'}if(!e.includes(" ")&&!e.includes("\t")&&!e.includes('"')){return e}if(!e.includes('"')&&!e.includes("\\")){return`"${e}"`}let t='"';let r=true;for(let n=e.length;n>0;n--){t+=e[n-1];if(r&&e[n-1]==="\\"){t+="\\"}else if(e[n-1]==='"'){r=true;t+="\\"}else{r=false}}t+='"';return t.split("").reverse().join("")}_cloneExecOptions(e){e=e||{};const t={cwd:e.cwd||process.cwd(),env:e.env||process.env,silent:e.silent||false,windowsVerbatimArguments:e.windowsVerbatimArguments||false,failOnStdErr:e.failOnStdErr||false,ignoreReturnCode:e.ignoreReturnCode||false,delay:e.delay||1e4};t.outStream=e.outStream||process.stdout;t.errStream=e.errStream||process.stderr;return t}_getSpawnOptions(e,t){e=e||{};const r={};r.cwd=e.cwd;r.env=e.env;r["windowsVerbatimArguments"]=e.windowsVerbatimArguments||this._isCmdFile();if(e.windowsVerbatimArguments){r.argv0=`"${t}"`}return r}exec(){return n(this,void 0,void 0,function*(){if(!l.isRooted(this.toolPath)&&(this.toolPath.includes("/")||f&&this.toolPath.includes("\\"))){this.toolPath=c.resolve(process.cwd(),this.options.cwd||process.cwd(),this.toolPath)}this.toolPath=yield u.which(this.toolPath,true);return new Promise((e,t)=>{this._debug(`exec tool: ${this.toolPath}`);this._debug("arguments:");for(const e of this.args){this._debug(` ${e}`)}const r=this._cloneExecOptions(this.options);if(!r.silent&&r.outStream){r.outStream.write(this._getCommandString(r)+o.EOL)}const n=new ExecState(r,this.toolPath);n.on("debug",e=>{this._debug(e)});const i=this._getSpawnFileName();const s=a.spawn(i,this._getSpawnArgs(r),this._getSpawnOptions(this.options,i));const c="";if(s.stdout){s.stdout.on("data",e=>{if(this.options.listeners&&this.options.listeners.stdout){this.options.listeners.stdout(e)}if(!r.silent&&r.outStream){r.outStream.write(e)}this._processLineBuffer(e,c,e=>{if(this.options.listeners&&this.options.listeners.stdline){this.options.listeners.stdline(e)}})})}const u="";if(s.stderr){s.stderr.on("data",e=>{n.processStderr=true;if(this.options.listeners&&this.options.listeners.stderr){this.options.listeners.stderr(e)}if(!r.silent&&r.errStream&&r.outStream){const t=r.failOnStdErr?r.errStream:r.outStream;t.write(e)}this._processLineBuffer(e,u,e=>{if(this.options.listeners&&this.options.listeners.errline){this.options.listeners.errline(e)}})})}s.on("error",e=>{n.processError=e.message;n.processExited=true;n.processClosed=true;n.CheckComplete()});s.on("exit",e=>{n.processExitCode=e;n.processExited=true;this._debug(`Exit code ${e} received from tool '${this.toolPath}'`);n.CheckComplete()});s.on("close",e=>{n.processExitCode=e;n.processExited=true;n.processClosed=true;this._debug(`STDIO streams have closed for tool '${this.toolPath}'`);n.CheckComplete()});n.on("done",(r,n)=>{if(c.length>0){this.emit("stdline",c)}if(u.length>0){this.emit("errline",u)}s.removeAllListeners();if(r){t(r)}else{e(n)}});if(this.options.input){if(!s.stdin){throw new Error("child process missing stdin")}s.stdin.end(this.options.input)}})})}}t.ToolRunner=ToolRunner;function argStringToArray(e){const t=[];let r=false;let n=false;let i="";function append(e){if(n&&e!=='"'){i+="\\"}i+=e;n=false}for(let o=0;o<e.length;o++){const s=e.charAt(o);if(s==='"'){if(!n){r=!r}else{append(s)}continue}if(s==="\\"&&n){append(s);continue}if(s==="\\"&&r){n=true;continue}if(s===" "&&!r){if(i.length>0){t.push(i);i=""}continue}append(s)}if(i.length>0){t.push(i.trim())}return t}t.argStringToArray=argStringToArray;class ExecState extends s.EventEmitter{constructor(e,t){super();this.processClosed=false;this.processError="";this.processExitCode=0;this.processExited=false;this.processStderr=false;this.delay=1e4;this.done=false;this.timeout=null;if(!t){throw new Error("toolPath must not be empty")}this.options=e;this.toolPath=t;if(e.delay){this.delay=e.delay}}CheckComplete(){if(this.done){return}if(this.processClosed){this._setResult()}else if(this.processExited){this.timeout=setTimeout(ExecState.HandleTimeout,this.delay,this)}}_debug(e){this.emit("debug",e)}_setResult(){let e;if(this.processExited){if(this.processError){e=new Error(`There was an error when attempting to execute the process '${this.toolPath}'. This may indicate the process failed to start. Error: ${this.processError}`)}else if(this.processExitCode!==0&&!this.options.ignoreReturnCode){e=new Error(`The process '${this.toolPath}' failed with exit code ${this.processExitCode}`)}else if(this.processStderr&&this.options.failOnStdErr){e=new Error(`The process '${this.toolPath}' failed because one or more lines were written to the STDERR stream`)}}if(this.timeout){clearTimeout(this.timeout);this.timeout=null}this.done=true;this.emit("done",e,this.processExitCode)}static HandleTimeout(e){if(e.done){return}if(!e.processClosed&&e.processExited){const t=`The STDIO streams did not close within ${e.delay/1e3} seconds of the exit event from process '${e.toolPath}'. This may indicate a child process inherited the STDIO streams and has not yet exited.`;e._debug(t)}e._setResult()}}},15:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__asyncValues||function(e){if(!Symbol.asyncIterator)throw new TypeError("Symbol.asyncIterator is not defined.");var t=e[Symbol.asyncIterator],r;return t?t.call(e):(e=typeof __values==="function"?__values(e):e[Symbol.iterator](),r={},verb("next"),verb("throw"),verb("return"),r[Symbol.asyncIterator]=function(){return this},r);function verb(t){r[t]=e[t]&&function(r){return new Promise(function(n,i){r=e[t](r),settle(n,i,r.done,r.value)})}}function settle(e,t,r,n){Promise.resolve(n).then(function(t){e({value:t,done:r})},t)}};var o=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const s=o(r(470));const a=o(r(986));const c=o(r(281));const u=o(r(1));const l=o(r(747));const f=o(r(622));const h=o(r(280));const p=o(r(669));const d=r(898);const v=r(931);function createTempDirectory(){return n(this,void 0,void 0,function*(){const e=process.platform==="win32";let t=process.env["RUNNER_TEMP"]||"";if(!t){let r;if(e){r=process.env["USERPROFILE"]||"C:\\"}else{if(process.platform==="darwin"){r="/Users"}else{r="/home"}}t=f.join(r,"actions","temp")}const r=f.join(t,d.v4());yield u.mkdirP(r);return r})}t.createTempDirectory=createTempDirectory;function getArchiveFileSizeIsBytes(e){return l.statSync(e).size}t.getArchiveFileSizeIsBytes=getArchiveFileSizeIsBytes;function resolvePaths(e){var t,r;var o;return n(this,void 0,void 0,function*(){const n=[];const a=(o=process.env["GITHUB_WORKSPACE"])!==null&&o!==void 0?o:process.cwd();const u=yield c.create(e.join("\n"),{implicitDescendants:false});try{for(var l=i(u.globGenerator()),h;h=yield l.next(),!h.done;){const e=h.value;const t=f.relative(a,e);s.debug(`Matched: ${t}`);n.push(`${t}`)}}catch(e){t={error:e}}finally{try{if(h&&!h.done&&(r=l.return))yield r.call(l)}finally{if(t)throw t.error}}return n})}t.resolvePaths=resolvePaths;function unlinkFile(e){return n(this,void 0,void 0,function*(){return p.promisify(l.unlink)(e)})}t.unlinkFile=unlinkFile;function getVersion(e){return n(this,void 0,void 0,function*(){s.debug(`Checking ${e} --version`);let t="";try{yield a.exec(`${e} --version`,[],{ignoreReturnCode:true,silent:true,listeners:{stdout:e=>t+=e.toString(),stderr:e=>t+=e.toString()}})}catch(e){s.debug(e.message)}t=t.trim();s.debug(t);return t})}function getCompressionMethod(){return n(this,void 0,void 0,function*(){if(process.platform==="win32"&&!(yield isGnuTarInstalled())){return v.CompressionMethod.Gzip}const e=yield getVersion("zstd");const t=h.clean(e);if(!e.toLowerCase().includes("zstd command line interface")){return v.CompressionMethod.Gzip}else if(!t||h.lt(t,"v1.3.2")){return v.CompressionMethod.ZstdWithoutLong}else{return v.CompressionMethod.Zstd}})}t.getCompressionMethod=getCompressionMethod;function getCacheFileName(e){return e===v.CompressionMethod.Gzip?v.CacheFilename.Gzip:v.CacheFilename.Zstd}t.getCacheFileName=getCacheFileName;function isGnuTarInstalled(){return n(this,void 0,void 0,function*(){const e=yield getVersion("tar");return e.toLowerCase().includes("gnu tar")})}t.isGnuTarInstalled=isGnuTarInstalled},16:function(e){e.exports=require("tls")},86:function(e,t,r){var n=r(139);var i=r(722);var o;var s;var a=0;var c=0;function v1(e,t,r){var u=t&&r||0;var l=t||[];e=e||{};var f=e.node||o;var h=e.clockseq!==undefined?e.clockseq:s;if(f==null||h==null){var p=n();if(f==null){f=o=[p[0]|1,p[1],p[2],p[3],p[4],p[5]]}if(h==null){h=s=(p[6]<<8|p[7])&16383}}var d=e.msecs!==undefined?e.msecs:(new Date).getTime();var v=e.nsecs!==undefined?e.nsecs:c+1;var y=d-a+(v-c)/1e4;if(y<0&&e.clockseq===undefined){h=h+1&16383}if((y<0||d>a)&&e.nsecs===undefined){v=0}if(v>=1e4){throw new Error("uuid.v1(): Can't create more than 10M uuids/sec")}a=d;c=v;s=h;d+=122192928e5;var g=((d&268435455)*1e4+v)%4294967296;l[u++]=g>>>24&255;l[u++]=g>>>16&255;l[u++]=g>>>8&255;l[u++]=g&255;var m=d/4294967296*1e4&268435455;l[u++]=m>>>8&255;l[u++]=m&255;l[u++]=m>>>24&15|16;l[u++]=m>>>16&255;l[u++]=h>>>8|128;l[u++]=h&255;for(var E=0;E<6;++E){l[u+E]=f[E]}return t?t:i(l)}e.exports=v1},87:function(e){e.exports=require("os")},93:function(e,t,r){e.exports=minimatch;minimatch.Minimatch=Minimatch;var n={sep:"/"};try{n=r(622)}catch(e){}var i=minimatch.GLOBSTAR=Minimatch.GLOBSTAR={};var o=r(306);var s={"!":{open:"(?:(?!(?:",close:"))[^/]*?)"},"?":{open:"(?:",close:")?"},"+":{open:"(?:",close:")+"},"*":{open:"(?:",close:")*"},"@":{open:"(?:",close:")"}};var a="[^/]";var c=a+"*?";var u="(?:(?!(?:\\/|^)(?:\\.{1,2})($|\\/)).)*?";var l="(?:(?!(?:\\/|^)\\.).)*?";var f=charSet("().*{}+?[]^$\\!");function charSet(e){return e.split("").reduce(function(e,t){e[t]=true;return e},{})}var h=/\/+/;minimatch.filter=filter;function filter(e,t){t=t||{};return function(r,n,i){return minimatch(r,e,t)}}function ext(e,t){e=e||{};t=t||{};var r={};Object.keys(t).forEach(function(e){r[e]=t[e]});Object.keys(e).forEach(function(t){r[t]=e[t]});return r}minimatch.defaults=function(e){if(!e||!Object.keys(e).length)return minimatch;var t=minimatch;var r=function minimatch(r,n,i){return t.minimatch(r,n,ext(e,i))};r.Minimatch=function Minimatch(r,n){return new t.Minimatch(r,ext(e,n))};return r};Minimatch.defaults=function(e){if(!e||!Object.keys(e).length)return Minimatch;return minimatch.defaults(e).Minimatch};function minimatch(e,t,r){if(typeof t!=="string"){throw new TypeError("glob pattern string required")}if(!r)r={};if(!r.nocomment&&t.charAt(0)==="#"){return false}if(t.trim()==="")return e==="";return new Minimatch(t,r).match(e)}function Minimatch(e,t){if(!(this instanceof Minimatch)){return new Minimatch(e,t)}if(typeof e!=="string"){throw new TypeError("glob pattern string required")}if(!t)t={};e=e.trim();if(n.sep!=="/"){e=e.split(n.sep).join("/")}this.options=t;this.set=[];this.pattern=e;this.regexp=null;this.negate=false;this.comment=false;this.empty=false;this.make()}Minimatch.prototype.debug=function(){};Minimatch.prototype.make=make;function make(){if(this._made)return;var e=this.pattern;var t=this.options;if(!t.nocomment&&e.charAt(0)==="#"){this.comment=true;return}if(!e){this.empty=true;return}this.parseNegate();var r=this.globSet=this.braceExpand();if(t.debug)this.debug=console.error;this.debug(this.pattern,r);r=this.globParts=r.map(function(e){return e.split(h)});this.debug(this.pattern,r);r=r.map(function(e,t,r){return e.map(this.parse,this)},this);this.debug(this.pattern,r);r=r.filter(function(e){return e.indexOf(false)===-1});this.debug(this.pattern,r);this.set=r}Minimatch.prototype.parseNegate=parseNegate;function parseNegate(){var e=this.pattern;var t=false;var r=this.options;var n=0;if(r.nonegate)return;for(var i=0,o=e.length;i<o&&e.charAt(i)==="!";i++){t=!t;n++}if(n)this.pattern=e.substr(n);this.negate=t}minimatch.braceExpand=function(e,t){return braceExpand(e,t)};Minimatch.prototype.braceExpand=braceExpand;function braceExpand(e,t){if(!t){if(this instanceof Minimatch){t=this.options}else{t={}}}e=typeof e==="undefined"?this.pattern:e;if(typeof e==="undefined"){throw new TypeError("undefined pattern")}if(t.nobrace||!e.match(/\{.*\}/)){return[e]}return o(e)}Minimatch.prototype.parse=parse;var p={};function parse(e,t){if(e.length>1024*64){throw new TypeError("pattern is too long")}var r=this.options;if(!r.noglobstar&&e==="**")return i;if(e==="")return"";var n="";var o=!!r.nocase;var u=false;var l=[];var h=[];var d;var v=false;var y=-1;var g=-1;var m=e.charAt(0)==="."?"":r.dot?"(?!(?:^|\\/)\\.{1,2}(?:$|\\/))":"(?!\\.)";var E=this;function clearStateChar(){if(d){switch(d){case"*":n+=c;o=true;break;case"?":n+=a;o=true;break;default:n+="\\"+d;break}E.debug("clearStateChar %j %j",d,n);d=false}}for(var S=0,R=e.length,w;S<R&&(w=e.charAt(S));S++){this.debug("%s\t%s %s %j",e,S,n,w);if(u&&f[w]){n+="\\"+w;u=false;continue}switch(w){case"/":return false;case"\\":clearStateChar();u=true;continue;case"?":case"*":case"+":case"@":case"!":this.debug("%s\t%s %s %j <-- stateChar",e,S,n,w);if(v){this.debug(" in class");if(w==="!"&&S===g+1)w="^";n+=w;continue}E.debug("call clearStateChar %j",d);clearStateChar();d=w;if(r.noext)clearStateChar();continue;case"(":if(v){n+="(";continue}if(!d){n+="\\(";continue}l.push({type:d,start:S-1,reStart:n.length,open:s[d].open,close:s[d].close});n+=d==="!"?"(?:(?!(?:":"(?:";this.debug("plType %j %j",d,n);d=false;continue;case")":if(v||!l.length){n+="\\)";continue}clearStateChar();o=true;var C=l.pop();n+=C.close;if(C.type==="!"){h.push(C)}C.reEnd=n.length;continue;case"|":if(v||!l.length||u){n+="\\|";u=false;continue}clearStateChar();n+="|";continue;case"[":clearStateChar();if(v){n+="\\"+w;continue}v=true;g=S;y=n.length;n+=w;continue;case"]":if(S===g+1||!v){n+="\\"+w;u=false;continue}if(v){var b=e.substring(g+1,S);try{RegExp("["+b+"]")}catch(e){var O=this.parse(b,p);n=n.substr(0,y)+"\\["+O[0]+"\\]";o=o||O[1];v=false;continue}}o=true;v=false;n+=w;continue;default:clearStateChar();if(u){u=false}else if(f[w]&&!(w==="^"&&v)){n+="\\"}n+=w}}if(v){b=e.substr(g+1);O=this.parse(b,p);n=n.substr(0,y)+"\\["+O[0];o=o||O[1]}for(C=l.pop();C;C=l.pop()){var A=n.slice(C.reStart+C.open.length);this.debug("setting tail",n,C);A=A.replace(/((?:\\{2}){0,64})(\\?)\|/g,function(e,t,r){if(!r){r="\\"}return t+t+r+"|"});this.debug("tail=%j\n %s",A,A,C,n);var T=C.type==="*"?c:C.type==="?"?a:"\\"+C.type;o=true;n=n.slice(0,C.reStart)+T+"\\("+A}clearStateChar();if(u){n+="\\\\"}var I=false;switch(n.charAt(0)){case".":case"[":case"(":I=true}for(var _=h.length-1;_>-1;_--){var x=h[_];var N=n.slice(0,x.reStart);var k=n.slice(x.reStart,x.reEnd-8);var L=n.slice(x.reEnd-8,x.reEnd);var P=n.slice(x.reEnd);L+=P;var $=N.split("(").length-1;var D=P;for(S=0;S<$;S++){D=D.replace(/\)[+*?]?/,"")}P=D;var j="";if(P===""&&t!==p){j="$"}var G=N+k+P+j+L;n=G}if(n!==""&&o){n="(?=.)"+n}if(I){n=m+n}if(t===p){return[n,o]}if(!o){return globUnescape(e)}var F=r.nocase?"i":"";try{var U=new RegExp("^"+n+"$",F)}catch(e){return new RegExp("$.")}U._glob=e;U._src=n;return U}minimatch.makeRe=function(e,t){return new Minimatch(e,t||{}).makeRe()};Minimatch.prototype.makeRe=makeRe;function makeRe(){if(this.regexp||this.regexp===false)return this.regexp;var e=this.set;if(!e.length){this.regexp=false;return this.regexp}var t=this.options;var r=t.noglobstar?c:t.dot?u:l;var n=t.nocase?"i":"";var o=e.map(function(e){return e.map(function(e){return e===i?r:typeof e==="string"?regExpEscape(e):e._src}).join("\\/")}).join("|");o="^(?:"+o+")$";if(this.negate)o="^(?!"+o+").*$";try{this.regexp=new RegExp(o,n)}catch(e){this.regexp=false}return this.regexp}minimatch.match=function(e,t,r){r=r||{};var n=new Minimatch(t,r);e=e.filter(function(e){return n.match(e)});if(n.options.nonull&&!e.length){e.push(t)}return e};Minimatch.prototype.match=match;function match(e,t){this.debug("match",e,this.pattern);if(this.comment)return false;if(this.empty)return e==="";if(e==="/"&&t)return true;var r=this.options;if(n.sep!=="/"){e=e.split(n.sep).join("/")}e=e.split(h);this.debug(this.pattern,"split",e);var i=this.set;this.debug(this.pattern,"set",i);var o;var s;for(s=e.length-1;s>=0;s--){o=e[s];if(o)break}for(s=0;s<i.length;s++){var a=i[s];var c=e;if(r.matchBase&&a.length===1){c=[o]}var u=this.matchOne(c,a,t);if(u){if(r.flipNegate)return true;return!this.negate}}if(r.flipNegate)return false;return this.negate}Minimatch.prototype.matchOne=function(e,t,r){var n=this.options;this.debug("matchOne",{this:this,file:e,pattern:t});this.debug("matchOne",e.length,t.length);for(var o=0,s=0,a=e.length,c=t.length;o<a&&s<c;o++,s++){this.debug("matchOne loop");var u=t[s];var l=e[o];this.debug(t,u,l);if(u===false)return false;if(u===i){this.debug("GLOBSTAR",[t,u,l]);var f=o;var h=s+1;if(h===c){this.debug("** at the end");for(;o<a;o++){if(e[o]==="."||e[o]===".."||!n.dot&&e[o].charAt(0)===".")return false}return true}while(f<a){var p=e[f];this.debug("\nglobstar while",e,f,t,h,p);if(this.matchOne(e.slice(f),t.slice(h),r)){this.debug("globstar found match!",f,a,p);return true}else{if(p==="."||p===".."||!n.dot&&p.charAt(0)==="."){this.debug("dot detected!",e,f,t,h);break}this.debug("globstar swallow a segment, and continue");f++}}if(r){this.debug("\n>>> no match, partial?",e,f,t,h);if(f===a)return true}return false}var d;if(typeof u==="string"){if(n.nocase){d=l.toLowerCase()===u.toLowerCase()}else{d=l===u}this.debug("string match",u,l,d)}else{d=l.match(u);this.debug("pattern match",u,l,d)}if(!d)return false}if(o===a&&s===c){return true}else if(o===a){return r}else if(s===c){var v=o===a-1&&e[o]==="";return v}throw new Error("wtf?")};function globUnescape(e){return e.replace(/\\(.)/g,"$1")}function regExpEscape(e){return e.replace(/[-[\]{}()*+?.,\\^$|#\s]/g,"\\$&")}},114:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=i(r(470));const s=r(539);const a=r(226);const c=i(r(417));const u=i(r(747));const l=i(r(794));const f=i(r(669));const h=i(r(15));const p=r(931);const d="1.0";function isSuccessStatusCode(e){if(!e){return false}return e>=200&&e<300}function isServerErrorStatusCode(e){if(!e){return true}return e>=500}function isRetryableStatusCode(e){if(!e){return false}const t=[s.HttpCodes.BadGateway,s.HttpCodes.ServiceUnavailable,s.HttpCodes.GatewayTimeout];return t.includes(e)}function getCacheApiUrl(e){const t=(process.env["ACTIONS_CACHE_URL"]||process.env["ACTIONS_RUNTIME_URL"]||"").replace("pipelines","artifactcache");if(!t){throw new Error("Cache Service Url not found, unable to restore cache.")}const r=`${t}_apis/artifactcache/${e}`;o.debug(`Resource Url: ${r}`);return r}function createAcceptHeader(e,t){return`${e};api-version=${t}`}function getRequestOptions(){const e={headers:{Accept:createAcceptHeader("application/json","6.0-preview.1")}};return e}function createHttpClient(){const e=process.env["ACTIONS_RUNTIME_TOKEN"]||"";const t=new a.BearerCredentialHandler(e);return new s.HttpClient("actions/cache",[t],getRequestOptions())}function getCacheVersion(e,t){const r=e.concat(!t||t===p.CompressionMethod.Gzip?[]:[t]);r.push(d);return c.createHash("sha256").update(r.join("|")).digest("hex")}t.getCacheVersion=getCacheVersion;function retry(e,t,r,i=2){return n(this,void 0,void 0,function*(){let n=undefined;let s=undefined;let a=false;let c="";let u=1;while(u<=i){try{n=yield t();s=r(n);if(!isServerErrorStatusCode(s)){return n}a=isRetryableStatusCode(s);c=`Cache service responded with ${s}`}catch(e){a=true;c=e.message}o.debug(`${e} - Attempt ${u} of ${i} failed with error: ${c}`);if(!a){o.debug(`${e} - Error is not retryable`);break}u++}throw Error(`${e} failed: ${c}`)})}t.retry=retry;function retryTypedResponse(e,t,r=2){return n(this,void 0,void 0,function*(){return yield retry(e,t,e=>e.statusCode,r)})}t.retryTypedResponse=retryTypedResponse;function retryHttpClientResponse(e,t,r=2){return n(this,void 0,void 0,function*(){return yield retry(e,t,e=>e.message.statusCode,r)})}t.retryHttpClientResponse=retryHttpClientResponse;function getCacheEntry(e,t,r){return n(this,void 0,void 0,function*(){const i=createHttpClient();const s=getCacheVersion(t,r===null||r===void 0?void 0:r.compressionMethod);const a=`cache?keys=${encodeURIComponent(e.join(","))}&version=${s}`;const c=yield retryTypedResponse("getCacheEntry",()=>n(this,void 0,void 0,function*(){return i.getJson(getCacheApiUrl(a))}));if(c.statusCode===204){return null}if(!isSuccessStatusCode(c.statusCode)){throw new Error(`Cache service responded with ${c.statusCode}`)}const u=c.result;const l=u===null||u===void 0?void 0:u.archiveLocation;if(!l){throw new Error("Cache not found.")}o.setSecret(l);o.debug(`Cache Result:`);o.debug(JSON.stringify(u));return u})}t.getCacheEntry=getCacheEntry;function pipeResponseToStream(e,t){return n(this,void 0,void 0,function*(){const r=f.promisify(l.pipeline);yield r(e.message,t)})}function downloadCache(e,t){return n(this,void 0,void 0,function*(){const r=u.createWriteStream(t);const i=new s.HttpClient("actions/cache");const a=yield retryHttpClientResponse("downloadCache",()=>n(this,void 0,void 0,function*(){return i.get(e)}));a.message.socket.setTimeout(p.SocketTimeout,()=>{a.message.destroy();o.debug(`Aborting download, socket timed out after ${p.SocketTimeout} ms`)});yield pipeResponseToStream(a,r);const c=a.message.headers["content-length"];if(c){const e=parseInt(c);const r=h.getArchiveFileSizeIsBytes(t);if(r!==e){throw new Error(`Incomplete download. Expected file size: ${e}, actual file size: ${r}`)}}else{o.debug("Unable to validate download, no Content-Length header")}})}t.downloadCache=downloadCache;function reserveCache(e,t,r){var i,o;return n(this,void 0,void 0,function*(){const s=createHttpClient();const a=getCacheVersion(t,r===null||r===void 0?void 0:r.compressionMethod);const c={key:e,version:a};const u=yield retryTypedResponse("reserveCache",()=>n(this,void 0,void 0,function*(){return s.postJson(getCacheApiUrl("caches"),c)}));return(o=(i=u===null||u===void 0?void 0:u.result)===null||i===void 0?void 0:i.cacheId)!==null&&o!==void 0?o:-1})}t.reserveCache=reserveCache;function getContentRange(e,t){return`bytes ${e}-${t}/*`}function uploadChunk(e,t,r,i,s){return n(this,void 0,void 0,function*(){o.debug(`Uploading chunk of size ${s-i+1} bytes at offset ${i} with content range: ${getContentRange(i,s)}`);const a={"Content-Type":"application/octet-stream","Content-Range":getContentRange(i,s)};yield retryHttpClientResponse(`uploadChunk (start: ${i}, end: ${s})`,()=>n(this,void 0,void 0,function*(){return e.sendStream("PATCH",t,r(),a)}))})}function uploadFile(e,t,r,i){var s,a;return n(this,void 0,void 0,function*(){const c=u.statSync(r).size;const l=getCacheApiUrl(`caches/${t.toString()}`);const f=u.openSync(r,"r");const h=(s=i===null||i===void 0?void 0:i.uploadConcurrency)!==null&&s!==void 0?s:4;const p=(a=i===null||i===void 0?void 0:i.uploadChunkSize)!==null&&a!==void 0?a:32*1024*1024;o.debug(`Concurrency: ${h} and Chunk Size: ${p}`);const d=[...new Array(h).keys()];o.debug("Awaiting all uploads");let v=0;try{yield Promise.all(d.map(()=>n(this,void 0,void 0,function*(){while(v<c){const t=Math.min(c-v,p);const n=v;const i=v+t-1;v+=p;yield uploadChunk(e,l,()=>u.createReadStream(r,{fd:f,start:n,end:i,autoClose:false}).on("error",e=>{throw new Error(`Cache upload failed because file read failed with ${e.Message}`)}),n,i)}})))}finally{u.closeSync(f)}return})}function commitCache(e,t,r){return n(this,void 0,void 0,function*(){const i={size:r};return yield retryTypedResponse("commitCache",()=>n(this,void 0,void 0,function*(){return e.postJson(getCacheApiUrl(`caches/${t.toString()}`),i)}))})}function saveCache(e,t,r){return n(this,void 0,void 0,function*(){const n=createHttpClient();o.debug("Upload cache");yield uploadFile(n,e,t,r);o.debug("Commiting cache");const i=h.getArchiveFileSizeIsBytes(t);const s=yield commitCache(n,e,i);if(!isSuccessStatusCode(s.statusCode)){throw new Error(`Cache service responded with ${s.statusCode} during commit cache.`)}o.info("Cache saved successfully")})}t.saveCache=saveCache},129:function(e){e.exports=require("child_process")},139:function(e,t,r){var n=r(417);e.exports=function nodeRNG(){return n.randomBytes(16)}},141:function(e,t,r){"use strict";var n=r(631);var i=r(16);var o=r(605);var s=r(211);var a=r(614);var c=r(357);var u=r(669);t.httpOverHttp=httpOverHttp;t.httpsOverHttp=httpsOverHttp;t.httpOverHttps=httpOverHttps;t.httpsOverHttps=httpsOverHttps;function httpOverHttp(e){var t=new TunnelingAgent(e);t.request=o.request;return t}function httpsOverHttp(e){var t=new TunnelingAgent(e);t.request=o.request;t.createSocket=createSecureSocket;t.defaultPort=443;return t}function httpOverHttps(e){var t=new TunnelingAgent(e);t.request=s.request;return t}function httpsOverHttps(e){var t=new TunnelingAgent(e);t.request=s.request;t.createSocket=createSecureSocket;t.defaultPort=443;return t}function TunnelingAgent(e){var t=this;t.options=e||{};t.proxyOptions=t.options.proxy||{};t.maxSockets=t.options.maxSockets||o.Agent.defaultMaxSockets;t.requests=[];t.sockets=[];t.on("free",function onFree(e,r,n,i){var o=toOptions(r,n,i);for(var s=0,a=t.requests.length;s<a;++s){var c=t.requests[s];if(c.host===o.host&&c.port===o.port){t.requests.splice(s,1);c.request.onSocket(e);return}}e.destroy();t.removeSocket(e)})}u.inherits(TunnelingAgent,a.EventEmitter);TunnelingAgent.prototype.addRequest=function addRequest(e,t,r,n){var i=this;var o=mergeOptions({request:e},i.options,toOptions(t,r,n));if(i.sockets.length>=this.maxSockets){i.requests.push(o);return}i.createSocket(o,function(t){t.on("free",onFree);t.on("close",onCloseOrRemove);t.on("agentRemove",onCloseOrRemove);e.onSocket(t);function onFree(){i.emit("free",t,o)}function onCloseOrRemove(e){i.removeSocket(t);t.removeListener("free",onFree);t.removeListener("close",onCloseOrRemove);t.removeListener("agentRemove",onCloseOrRemove)}})};TunnelingAgent.prototype.createSocket=function createSocket(e,t){var r=this;var n={};r.sockets.push(n);var i=mergeOptions({},r.proxyOptions,{method:"CONNECT",path:e.host+":"+e.port,agent:false,headers:{host:e.host+":"+e.port}});if(e.localAddress){i.localAddress=e.localAddress}if(i.proxyAuth){i.headers=i.headers||{};i.headers["Proxy-Authorization"]="Basic "+new Buffer(i.proxyAuth).toString("base64")}l("making CONNECT request");var o=r.request(i);o.useChunkedEncodingByDefault=false;o.once("response",onResponse);o.once("upgrade",onUpgrade);o.once("connect",onConnect);o.once("error",onError);o.end();function onResponse(e){e.upgrade=true}function onUpgrade(e,t,r){process.nextTick(function(){onConnect(e,t,r)})}function onConnect(i,s,a){o.removeAllListeners();s.removeAllListeners();if(i.statusCode!==200){l("tunneling socket could not be established, statusCode=%d",i.statusCode);s.destroy();var c=new Error("tunneling socket could not be established, "+"statusCode="+i.statusCode);c.code="ECONNRESET";e.request.emit("error",c);r.removeSocket(n);return}if(a.length>0){l("got illegal response body from proxy");s.destroy();var c=new Error("got illegal response body from proxy");c.code="ECONNRESET";e.request.emit("error",c);r.removeSocket(n);return}l("tunneling connection has established");r.sockets[r.sockets.indexOf(n)]=s;return t(s)}function onError(t){o.removeAllListeners();l("tunneling socket could not be established, cause=%s\n",t.message,t.stack);var i=new Error("tunneling socket could not be established, "+"cause="+t.message);i.code="ECONNRESET";e.request.emit("error",i);r.removeSocket(n)}};TunnelingAgent.prototype.removeSocket=function removeSocket(e){var t=this.sockets.indexOf(e);if(t===-1){return}this.sockets.splice(t,1);var r=this.requests.shift();if(r){this.createSocket(r,function(e){r.request.onSocket(e)})}};function createSecureSocket(e,t){var r=this;TunnelingAgent.prototype.createSocket.call(r,e,function(n){var o=e.request.getHeader("host");var s=mergeOptions({},r.options,{socket:n,servername:o?o.replace(/:.*$/,""):e.host});var a=i.connect(0,s);r.sockets[r.sockets.indexOf(n)]=a;t(a)})}function toOptions(e,t,r){if(typeof e==="string"){return{host:e,port:t,localAddress:r}}return e}function mergeOptions(e){for(var t=1,r=arguments.length;t<r;++t){var n=arguments[t];if(typeof n==="object"){var i=Object.keys(n);for(var o=0,s=i.length;o<s;++o){var a=i[o];if(n[a]!==undefined){e[a]=n[a]}}}}return e}var l;if(process.env.NODE_DEBUG&&/\btunnel\b/.test(process.env.NODE_DEBUG)){l=function(){var e=Array.prototype.slice.call(arguments);if(typeof e[0]==="string"){e[0]="TUNNEL: "+e[0]}else{e.unshift("TUNNEL:")}console.error.apply(console,e)}}else{l=function(){}}t.debug=l},167:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=i(r(622));const s=i(r(747));const a=i(r(87));const c=i(r(470));const u=i(r(692));const l=i(r(888));const f=i(r(662));const h="DEPENDENCIES_CACHE_PATH";const p="DEPENDENCIES_CACHE_KEY";const d="DEPENDENCIES_CACHE_RESULT";function restoreCachedDependencies(e){return n(this,void 0,void 0,function*(){const t=o.resolve(a.homedir(),".gradle/caches/modules-2");c.saveState(h,t);const r=l.inputArrayOrNull("dependencies-cache-key");const n=r?r:["**/*.gradle","**/*.gradle.kts","**/gradle.properties","gradle/**"];const i=yield f.hashFiles(e,n);const s="dependencies-";const v=`${s}${i}`;c.saveState(p,v);const y=yield u.restoreCache([t],v,[s]);c.saveState(d,y)})}t.restoreCachedDependencies=restoreCachedDependencies;function cacheDependencies(){return n(this,void 0,void 0,function*(){const e=c.getState(h);const t=c.getState(p);const r=c.getState(d);if(!e||!s.existsSync(e)){c.debug("No dependencies to cache.");return}if(r&&t===r){c.info(`Dependencies cache hit occurred on the cache key ${t}, not saving cache.`);return}try{yield u.saveCache([e],t)}catch(e){if(e.name===u.ValidationError.name){throw e}else if(e.name===u.ReserveCacheError.name){c.info(e.message)}else{c.info(`[warning] ${e.message}`)}}return})}t.cacheDependencies=cacheDependencies},211:function(e){e.exports=require("https")},226:function(e,t){"use strict";Object.defineProperty(t,"__esModule",{value:true});class BasicCredentialHandler{constructor(e,t){this.username=e;this.password=t}prepareRequest(e){e.headers["Authorization"]="Basic "+Buffer.from(this.username+":"+this.password).toString("base64")}canHandleAuthentication(e){return false}handleAuthentication(e,t,r){return null}}t.BasicCredentialHandler=BasicCredentialHandler;class BearerCredentialHandler{constructor(e){this.token=e}prepareRequest(e){e.headers["Authorization"]="Bearer "+this.token}canHandleAuthentication(e){return false}handleAuthentication(e,t,r){return null}}t.BearerCredentialHandler=BearerCredentialHandler;class PersonalAccessTokenCredentialHandler{constructor(e){this.token=e}prepareRequest(e){e.headers["Authorization"]="Basic "+Buffer.from("PAT:"+this.token).toString("base64")}canHandleAuthentication(e){return false}handleAuthentication(e,t,r){return null}}t.PersonalAccessTokenCredentialHandler=PersonalAccessTokenCredentialHandler},280:function(e,t){t=e.exports=SemVer;var r;if(typeof process==="object"&&process.env&&process.env.NODE_DEBUG&&/\bsemver\b/i.test(process.env.NODE_DEBUG)){r=function(){var e=Array.prototype.slice.call(arguments,0);e.unshift("SEMVER");console.log.apply(console,e)}}else{r=function(){}}t.SEMVER_SPEC_VERSION="2.0.0";var n=256;var i=Number.MAX_SAFE_INTEGER||9007199254740991;var o=16;var s=t.re=[];var a=t.src=[];var c=t.tokens={};var u=0;function tok(e){c[e]=u++}tok("NUMERICIDENTIFIER");a[c.NUMERICIDENTIFIER]="0|[1-9]\\d*";tok("NUMERICIDENTIFIERLOOSE");a[c.NUMERICIDENTIFIERLOOSE]="[0-9]+";tok("NONNUMERICIDENTIFIER");a[c.NONNUMERICIDENTIFIER]="\\d*[a-zA-Z-][a-zA-Z0-9-]*";tok("MAINVERSION");a[c.MAINVERSION]="("+a[c.NUMERICIDENTIFIER]+")\\."+"("+a[c.NUMERICIDENTIFIER]+")\\."+"("+a[c.NUMERICIDENTIFIER]+")";tok("MAINVERSIONLOOSE");a[c.MAINVERSIONLOOSE]="("+a[c.NUMERICIDENTIFIERLOOSE]+")\\."+"("+a[c.NUMERICIDENTIFIERLOOSE]+")\\."+"("+a[c.NUMERICIDENTIFIERLOOSE]+")";tok("PRERELEASEIDENTIFIER");a[c.PRERELEASEIDENTIFIER]="(?:"+a[c.NUMERICIDENTIFIER]+"|"+a[c.NONNUMERICIDENTIFIER]+")";tok("PRERELEASEIDENTIFIERLOOSE");a[c.PRERELEASEIDENTIFIERLOOSE]="(?:"+a[c.NUMERICIDENTIFIERLOOSE]+"|"+a[c.NONNUMERICIDENTIFIER]+")";tok("PRERELEASE");a[c.PRERELEASE]="(?:-("+a[c.PRERELEASEIDENTIFIER]+"(?:\\."+a[c.PRERELEASEIDENTIFIER]+")*))";tok("PRERELEASELOOSE");a[c.PRERELEASELOOSE]="(?:-?("+a[c.PRERELEASEIDENTIFIERLOOSE]+"(?:\\."+a[c.PRERELEASEIDENTIFIERLOOSE]+")*))";tok("BUILDIDENTIFIER");a[c.BUILDIDENTIFIER]="[0-9A-Za-z-]+";tok("BUILD");a[c.BUILD]="(?:\\+("+a[c.BUILDIDENTIFIER]+"(?:\\."+a[c.BUILDIDENTIFIER]+")*))";tok("FULL");tok("FULLPLAIN");a[c.FULLPLAIN]="v?"+a[c.MAINVERSION]+a[c.PRERELEASE]+"?"+a[c.BUILD]+"?";a[c.FULL]="^"+a[c.FULLPLAIN]+"$";tok("LOOSEPLAIN");a[c.LOOSEPLAIN]="[v=\\s]*"+a[c.MAINVERSIONLOOSE]+a[c.PRERELEASELOOSE]+"?"+a[c.BUILD]+"?";tok("LOOSE");a[c.LOOSE]="^"+a[c.LOOSEPLAIN]+"$";tok("GTLT");a[c.GTLT]="((?:<|>)?=?)";tok("XRANGEIDENTIFIERLOOSE");a[c.XRANGEIDENTIFIERLOOSE]=a[c.NUMERICIDENTIFIERLOOSE]+"|x|X|\\*";tok("XRANGEIDENTIFIER");a[c.XRANGEIDENTIFIER]=a[c.NUMERICIDENTIFIER]+"|x|X|\\*";tok("XRANGEPLAIN");a[c.XRANGEPLAIN]="[v=\\s]*("+a[c.XRANGEIDENTIFIER]+")"+"(?:\\.("+a[c.XRANGEIDENTIFIER]+")"+"(?:\\.("+a[c.XRANGEIDENTIFIER]+")"+"(?:"+a[c.PRERELEASE]+")?"+a[c.BUILD]+"?"+")?)?";tok("XRANGEPLAINLOOSE");a[c.XRANGEPLAINLOOSE]="[v=\\s]*("+a[c.XRANGEIDENTIFIERLOOSE]+")"+"(?:\\.("+a[c.XRANGEIDENTIFIERLOOSE]+")"+"(?:\\.("+a[c.XRANGEIDENTIFIERLOOSE]+")"+"(?:"+a[c.PRERELEASELOOSE]+")?"+a[c.BUILD]+"?"+")?)?";tok("XRANGE");a[c.XRANGE]="^"+a[c.GTLT]+"\\s*"+a[c.XRANGEPLAIN]+"$";tok("XRANGELOOSE");a[c.XRANGELOOSE]="^"+a[c.GTLT]+"\\s*"+a[c.XRANGEPLAINLOOSE]+"$";tok("COERCE");a[c.COERCE]="(^|[^\\d])"+"(\\d{1,"+o+"})"+"(?:\\.(\\d{1,"+o+"}))?"+"(?:\\.(\\d{1,"+o+"}))?"+"(?:$|[^\\d])";tok("COERCERTL");s[c.COERCERTL]=new RegExp(a[c.COERCE],"g");tok("LONETILDE");a[c.LONETILDE]="(?:~>?)";tok("TILDETRIM");a[c.TILDETRIM]="(\\s*)"+a[c.LONETILDE]+"\\s+";s[c.TILDETRIM]=new RegExp(a[c.TILDETRIM],"g");var l="$1~";tok("TILDE");a[c.TILDE]="^"+a[c.LONETILDE]+a[c.XRANGEPLAIN]+"$";tok("TILDELOOSE");a[c.TILDELOOSE]="^"+a[c.LONETILDE]+a[c.XRANGEPLAINLOOSE]+"$";tok("LONECARET");a[c.LONECARET]="(?:\\^)";tok("CARETTRIM");a[c.CARETTRIM]="(\\s*)"+a[c.LONECARET]+"\\s+";s[c.CARETTRIM]=new RegExp(a[c.CARETTRIM],"g");var f="$1^";tok("CARET");a[c.CARET]="^"+a[c.LONECARET]+a[c.XRANGEPLAIN]+"$";tok("CARETLOOSE");a[c.CARETLOOSE]="^"+a[c.LONECARET]+a[c.XRANGEPLAINLOOSE]+"$";tok("COMPARATORLOOSE");a[c.COMPARATORLOOSE]="^"+a[c.GTLT]+"\\s*("+a[c.LOOSEPLAIN]+")$|^$";tok("COMPARATOR");a[c.COMPARATOR]="^"+a[c.GTLT]+"\\s*("+a[c.FULLPLAIN]+")$|^$";tok("COMPARATORTRIM");a[c.COMPARATORTRIM]="(\\s*)"+a[c.GTLT]+"\\s*("+a[c.LOOSEPLAIN]+"|"+a[c.XRANGEPLAIN]+")";s[c.COMPARATORTRIM]=new RegExp(a[c.COMPARATORTRIM],"g");var h="$1$2$3";tok("HYPHENRANGE");a[c.HYPHENRANGE]="^\\s*("+a[c.XRANGEPLAIN]+")"+"\\s+-\\s+"+"("+a[c.XRANGEPLAIN]+")"+"\\s*$";tok("HYPHENRANGELOOSE");a[c.HYPHENRANGELOOSE]="^\\s*("+a[c.XRANGEPLAINLOOSE]+")"+"\\s+-\\s+"+"("+a[c.XRANGEPLAINLOOSE]+")"+"\\s*$";tok("STAR");a[c.STAR]="(<|>)?=?\\s*\\*";for(var p=0;p<u;p++){r(p,a[p]);if(!s[p]){s[p]=new RegExp(a[p])}}t.parse=parse;function parse(e,t){if(!t||typeof t!=="object"){t={loose:!!t,includePrerelease:false}}if(e instanceof SemVer){return e}if(typeof e!=="string"){return null}if(e.length>n){return null}var r=t.loose?s[c.LOOSE]:s[c.FULL];if(!r.test(e)){return null}try{return new SemVer(e,t)}catch(e){return null}}t.valid=valid;function valid(e,t){var r=parse(e,t);return r?r.version:null}t.clean=clean;function clean(e,t){var r=parse(e.trim().replace(/^[=v]+/,""),t);return r?r.version:null}t.SemVer=SemVer;function SemVer(e,t){if(!t||typeof t!=="object"){t={loose:!!t,includePrerelease:false}}if(e instanceof SemVer){if(e.loose===t.loose){return e}else{e=e.version}}else if(typeof e!=="string"){throw new TypeError("Invalid Version: "+e)}if(e.length>n){throw new TypeError("version is longer than "+n+" characters")}if(!(this instanceof SemVer)){return new SemVer(e,t)}r("SemVer",e,t);this.options=t;this.loose=!!t.loose;var o=e.trim().match(t.loose?s[c.LOOSE]:s[c.FULL]);if(!o){throw new TypeError("Invalid Version: "+e)}this.raw=e;this.major=+o[1];this.minor=+o[2];this.patch=+o[3];if(this.major>i||this.major<0){throw new TypeError("Invalid major version")}if(this.minor>i||this.minor<0){throw new TypeError("Invalid minor version")}if(this.patch>i||this.patch<0){throw new TypeError("Invalid patch version")}if(!o[4]){this.prerelease=[]}else{this.prerelease=o[4].split(".").map(function(e){if(/^[0-9]+$/.test(e)){var t=+e;if(t>=0&&t<i){return t}}return e})}this.build=o[5]?o[5].split("."):[];this.format()}SemVer.prototype.format=function(){this.version=this.major+"."+this.minor+"."+this.patch;if(this.prerelease.length){this.version+="-"+this.prerelease.join(".")}return this.version};SemVer.prototype.toString=function(){return this.version};SemVer.prototype.compare=function(e){r("SemVer.compare",this.version,this.options,e);if(!(e instanceof SemVer)){e=new SemVer(e,this.options)}return this.compareMain(e)||this.comparePre(e)};SemVer.prototype.compareMain=function(e){if(!(e instanceof SemVer)){e=new SemVer(e,this.options)}return compareIdentifiers(this.major,e.major)||compareIdentifiers(this.minor,e.minor)||compareIdentifiers(this.patch,e.patch)};SemVer.prototype.comparePre=function(e){if(!(e instanceof SemVer)){e=new SemVer(e,this.options)}if(this.prerelease.length&&!e.prerelease.length){return-1}else if(!this.prerelease.length&&e.prerelease.length){return 1}else if(!this.prerelease.length&&!e.prerelease.length){return 0}var t=0;do{var n=this.prerelease[t];var i=e.prerelease[t];r("prerelease compare",t,n,i);if(n===undefined&&i===undefined){return 0}else if(i===undefined){return 1}else if(n===undefined){return-1}else if(n===i){continue}else{return compareIdentifiers(n,i)}}while(++t)};SemVer.prototype.compareBuild=function(e){if(!(e instanceof SemVer)){e=new SemVer(e,this.options)}var t=0;do{var n=this.build[t];var i=e.build[t];r("prerelease compare",t,n,i);if(n===undefined&&i===undefined){return 0}else if(i===undefined){return 1}else if(n===undefined){return-1}else if(n===i){continue}else{return compareIdentifiers(n,i)}}while(++t)};SemVer.prototype.inc=function(e,t){switch(e){case"premajor":this.prerelease.length=0;this.patch=0;this.minor=0;this.major++;this.inc("pre",t);break;case"preminor":this.prerelease.length=0;this.patch=0;this.minor++;this.inc("pre",t);break;case"prepatch":this.prerelease.length=0;this.inc("patch",t);this.inc("pre",t);break;case"prerelease":if(this.prerelease.length===0){this.inc("patch",t)}this.inc("pre",t);break;case"major":if(this.minor!==0||this.patch!==0||this.prerelease.length===0){this.major++}this.minor=0;this.patch=0;this.prerelease=[];break;case"minor":if(this.patch!==0||this.prerelease.length===0){this.minor++}this.patch=0;this.prerelease=[];break;case"patch":if(this.prerelease.length===0){this.patch++}this.prerelease=[];break;case"pre":if(this.prerelease.length===0){this.prerelease=[0]}else{var r=this.prerelease.length;while(--r>=0){if(typeof this.prerelease[r]==="number"){this.prerelease[r]++;r=-2}}if(r===-1){this.prerelease.push(0)}}if(t){if(this.prerelease[0]===t){if(isNaN(this.prerelease[1])){this.prerelease=[t,0]}}else{this.prerelease=[t,0]}}break;default:throw new Error("invalid increment argument: "+e)}this.format();this.raw=this.version;return this};t.inc=inc;function inc(e,t,r,n){if(typeof r==="string"){n=r;r=undefined}try{return new SemVer(e,r).inc(t,n).version}catch(e){return null}}t.diff=diff;function diff(e,t){if(eq(e,t)){return null}else{var r=parse(e);var n=parse(t);var i="";if(r.prerelease.length||n.prerelease.length){i="pre";var o="prerelease"}for(var s in r){if(s==="major"||s==="minor"||s==="patch"){if(r[s]!==n[s]){return i+s}}}return o}}t.compareIdentifiers=compareIdentifiers;var d=/^[0-9]+$/;function compareIdentifiers(e,t){var r=d.test(e);var n=d.test(t);if(r&&n){e=+e;t=+t}return e===t?0:r&&!n?-1:n&&!r?1:e<t?-1:1}t.rcompareIdentifiers=rcompareIdentifiers;function rcompareIdentifiers(e,t){return compareIdentifiers(t,e)}t.major=major;function major(e,t){return new SemVer(e,t).major}t.minor=minor;function minor(e,t){return new SemVer(e,t).minor}t.patch=patch;function patch(e,t){return new SemVer(e,t).patch}t.compare=compare;function compare(e,t,r){return new SemVer(e,r).compare(new SemVer(t,r))}t.compareLoose=compareLoose;function compareLoose(e,t){return compare(e,t,true)}t.compareBuild=compareBuild;function compareBuild(e,t,r){var n=new SemVer(e,r);var i=new SemVer(t,r);return n.compare(i)||n.compareBuild(i)}t.rcompare=rcompare;function rcompare(e,t,r){return compare(t,e,r)}t.sort=sort;function sort(e,r){return e.sort(function(e,n){return t.compareBuild(e,n,r)})}t.rsort=rsort;function rsort(e,r){return e.sort(function(e,n){return t.compareBuild(n,e,r)})}t.gt=gt;function gt(e,t,r){return compare(e,t,r)>0}t.lt=lt;function lt(e,t,r){return compare(e,t,r)<0}t.eq=eq;function eq(e,t,r){return compare(e,t,r)===0}t.neq=neq;function neq(e,t,r){return compare(e,t,r)!==0}t.gte=gte;function gte(e,t,r){return compare(e,t,r)>=0}t.lte=lte;function lte(e,t,r){return compare(e,t,r)<=0}t.cmp=cmp;function cmp(e,t,r,n){switch(t){case"===":if(typeof e==="object")e=e.version;if(typeof r==="object")r=r.version;return e===r;case"!==":if(typeof e==="object")e=e.version;if(typeof r==="object")r=r.version;return e!==r;case"":case"=":case"==":return eq(e,r,n);case"!=":return neq(e,r,n);case">":return gt(e,r,n);case">=":return gte(e,r,n);case"<":return lt(e,r,n);case"<=":return lte(e,r,n);default:throw new TypeError("Invalid operator: "+t)}}t.Comparator=Comparator;function Comparator(e,t){if(!t||typeof t!=="object"){t={loose:!!t,includePrerelease:false}}if(e instanceof Comparator){if(e.loose===!!t.loose){return e}else{e=e.value}}if(!(this instanceof Comparator)){return new Comparator(e,t)}r("comparator",e,t);this.options=t;this.loose=!!t.loose;this.parse(e);if(this.semver===v){this.value=""}else{this.value=this.operator+this.semver.version}r("comp",this)}var v={};Comparator.prototype.parse=function(e){var t=this.options.loose?s[c.COMPARATORLOOSE]:s[c.COMPARATOR];var r=e.match(t);if(!r){throw new TypeError("Invalid comparator: "+e)}this.operator=r[1]!==undefined?r[1]:"";if(this.operator==="="){this.operator=""}if(!r[2]){this.semver=v}else{this.semver=new SemVer(r[2],this.options.loose)}};Comparator.prototype.toString=function(){return this.value};Comparator.prototype.test=function(e){r("Comparator.test",e,this.options.loose);if(this.semver===v||e===v){return true}if(typeof e==="string"){try{e=new SemVer(e,this.options)}catch(e){return false}}return cmp(e,this.operator,this.semver,this.options)};Comparator.prototype.intersects=function(e,t){if(!(e instanceof Comparator)){throw new TypeError("a Comparator is required")}if(!t||typeof t!=="object"){t={loose:!!t,includePrerelease:false}}var r;if(this.operator===""){if(this.value===""){return true}r=new Range(e.value,t);return satisfies(this.value,r,t)}else if(e.operator===""){if(e.value===""){return true}r=new Range(this.value,t);return satisfies(e.semver,r,t)}var n=(this.operator===">="||this.operator===">")&&(e.operator===">="||e.operator===">");var i=(this.operator==="<="||this.operator==="<")&&(e.operator==="<="||e.operator==="<");var o=this.semver.version===e.semver.version;var s=(this.operator===">="||this.operator==="<=")&&(e.operator===">="||e.operator==="<=");var a=cmp(this.semver,"<",e.semver,t)&&((this.operator===">="||this.operator===">")&&(e.operator==="<="||e.operator==="<"));var c=cmp(this.semver,">",e.semver,t)&&((this.operator==="<="||this.operator==="<")&&(e.operator===">="||e.operator===">"));return n||i||o&&s||a||c};t.Range=Range;function Range(e,t){if(!t||typeof t!=="object"){t={loose:!!t,includePrerelease:false}}if(e instanceof Range){if(e.loose===!!t.loose&&e.includePrerelease===!!t.includePrerelease){return e}else{return new Range(e.raw,t)}}if(e instanceof Comparator){return new Range(e.value,t)}if(!(this instanceof Range)){return new Range(e,t)}this.options=t;this.loose=!!t.loose;this.includePrerelease=!!t.includePrerelease;this.raw=e;this.set=e.split(/\s*\|\|\s*/).map(function(e){return this.parseRange(e.trim())},this).filter(function(e){return e.length});if(!this.set.length){throw new TypeError("Invalid SemVer Range: "+e)}this.format()}Range.prototype.format=function(){this.range=this.set.map(function(e){return e.join(" ").trim()}).join("||").trim();return this.range};Range.prototype.toString=function(){return this.range};Range.prototype.parseRange=function(e){var t=this.options.loose;e=e.trim();var n=t?s[c.HYPHENRANGELOOSE]:s[c.HYPHENRANGE];e=e.replace(n,hyphenReplace);r("hyphen replace",e);e=e.replace(s[c.COMPARATORTRIM],h);r("comparator trim",e,s[c.COMPARATORTRIM]);e=e.replace(s[c.TILDETRIM],l);e=e.replace(s[c.CARETTRIM],f);e=e.split(/\s+/).join(" ");var i=t?s[c.COMPARATORLOOSE]:s[c.COMPARATOR];var o=e.split(" ").map(function(e){return parseComparator(e,this.options)},this).join(" ").split(/\s+/);if(this.options.loose){o=o.filter(function(e){return!!e.match(i)})}o=o.map(function(e){return new Comparator(e,this.options)},this);return o};Range.prototype.intersects=function(e,t){if(!(e instanceof Range)){throw new TypeError("a Range is required")}return this.set.some(function(r){return isSatisfiable(r,t)&&e.set.some(function(e){return isSatisfiable(e,t)&&r.every(function(r){return e.every(function(e){return r.intersects(e,t)})})})})};function isSatisfiable(e,t){var r=true;var n=e.slice();var i=n.pop();while(r&&n.length){r=n.every(function(e){return i.intersects(e,t)});i=n.pop()}return r}t.toComparators=toComparators;function toComparators(e,t){return new Range(e,t).set.map(function(e){return e.map(function(e){return e.value}).join(" ").trim().split(" ")})}function parseComparator(e,t){r("comp",e,t);e=replaceCarets(e,t);r("caret",e);e=replaceTildes(e,t);r("tildes",e);e=replaceXRanges(e,t);r("xrange",e);e=replaceStars(e,t);r("stars",e);return e}function isX(e){return!e||e.toLowerCase()==="x"||e==="*"}function replaceTildes(e,t){return e.trim().split(/\s+/).map(function(e){return replaceTilde(e,t)}).join(" ")}function replaceTilde(e,t){var n=t.loose?s[c.TILDELOOSE]:s[c.TILDE];return e.replace(n,function(t,n,i,o,s){r("tilde",e,t,n,i,o,s);var a;if(isX(n)){a=""}else if(isX(i)){a=">="+n+".0.0 <"+(+n+1)+".0.0"}else if(isX(o)){a=">="+n+"."+i+".0 <"+n+"."+(+i+1)+".0"}else if(s){r("replaceTilde pr",s);a=">="+n+"."+i+"."+o+"-"+s+" <"+n+"."+(+i+1)+".0"}else{a=">="+n+"."+i+"."+o+" <"+n+"."+(+i+1)+".0"}r("tilde return",a);return a})}function replaceCarets(e,t){return e.trim().split(/\s+/).map(function(e){return replaceCaret(e,t)}).join(" ")}function replaceCaret(e,t){r("caret",e,t);var n=t.loose?s[c.CARETLOOSE]:s[c.CARET];return e.replace(n,function(t,n,i,o,s){r("caret",e,t,n,i,o,s);var a;if(isX(n)){a=""}else if(isX(i)){a=">="+n+".0.0 <"+(+n+1)+".0.0"}else if(isX(o)){if(n==="0"){a=">="+n+"."+i+".0 <"+n+"."+(+i+1)+".0"}else{a=">="+n+"."+i+".0 <"+(+n+1)+".0.0"}}else if(s){r("replaceCaret pr",s);if(n==="0"){if(i==="0"){a=">="+n+"."+i+"."+o+"-"+s+" <"+n+"."+i+"."+(+o+1)}else{a=">="+n+"."+i+"."+o+"-"+s+" <"+n+"."+(+i+1)+".0"}}else{a=">="+n+"."+i+"."+o+"-"+s+" <"+(+n+1)+".0.0"}}else{r("no pr");if(n==="0"){if(i==="0"){a=">="+n+"."+i+"."+o+" <"+n+"."+i+"."+(+o+1)}else{a=">="+n+"."+i+"."+o+" <"+n+"."+(+i+1)+".0"}}else{a=">="+n+"."+i+"."+o+" <"+(+n+1)+".0.0"}}r("caret return",a);return a})}function replaceXRanges(e,t){r("replaceXRanges",e,t);return e.split(/\s+/).map(function(e){return replaceXRange(e,t)}).join(" ")}function replaceXRange(e,t){e=e.trim();var n=t.loose?s[c.XRANGELOOSE]:s[c.XRANGE];return e.replace(n,function(n,i,o,s,a,c){r("xRange",e,n,i,o,s,a,c);var u=isX(o);var l=u||isX(s);var f=l||isX(a);var h=f;if(i==="="&&h){i=""}c=t.includePrerelease?"-0":"";if(u){if(i===">"||i==="<"){n="<0.0.0-0"}else{n="*"}}else if(i&&h){if(l){s=0}a=0;if(i===">"){i=">=";if(l){o=+o+1;s=0;a=0}else{s=+s+1;a=0}}else if(i==="<="){i="<";if(l){o=+o+1}else{s=+s+1}}n=i+o+"."+s+"."+a+c}else if(l){n=">="+o+".0.0"+c+" <"+(+o+1)+".0.0"+c}else if(f){n=">="+o+"."+s+".0"+c+" <"+o+"."+(+s+1)+".0"+c}r("xRange return",n);return n})}function replaceStars(e,t){r("replaceStars",e,t);return e.trim().replace(s[c.STAR],"")}function hyphenReplace(e,t,r,n,i,o,s,a,c,u,l,f,h){if(isX(r)){t=""}else if(isX(n)){t=">="+r+".0.0"}else if(isX(i)){t=">="+r+"."+n+".0"}else{t=">="+t}if(isX(c)){a=""}else if(isX(u)){a="<"+(+c+1)+".0.0"}else if(isX(l)){a="<"+c+"."+(+u+1)+".0"}else if(f){a="<="+c+"."+u+"."+l+"-"+f}else{a="<="+a}return(t+" "+a).trim()}Range.prototype.test=function(e){if(!e){return false}if(typeof e==="string"){try{e=new SemVer(e,this.options)}catch(e){return false}}for(var t=0;t<this.set.length;t++){if(testSet(this.set[t],e,this.options)){return true}}return false};function testSet(e,t,n){for(var i=0;i<e.length;i++){if(!e[i].test(t)){return false}}if(t.prerelease.length&&!n.includePrerelease){for(i=0;i<e.length;i++){r(e[i].semver);if(e[i].semver===v){continue}if(e[i].semver.prerelease.length>0){var o=e[i].semver;if(o.major===t.major&&o.minor===t.minor&&o.patch===t.patch){return true}}}return false}return true}t.satisfies=satisfies;function satisfies(e,t,r){try{t=new Range(t,r)}catch(e){return false}return t.test(e)}t.maxSatisfying=maxSatisfying;function maxSatisfying(e,t,r){var n=null;var i=null;try{var o=new Range(t,r)}catch(e){return null}e.forEach(function(e){if(o.test(e)){if(!n||i.compare(e)===-1){n=e;i=new SemVer(n,r)}}});return n}t.minSatisfying=minSatisfying;function minSatisfying(e,t,r){var n=null;var i=null;try{var o=new Range(t,r)}catch(e){return null}e.forEach(function(e){if(o.test(e)){if(!n||i.compare(e)===1){n=e;i=new SemVer(n,r)}}});return n}t.minVersion=minVersion;function minVersion(e,t){e=new Range(e,t);var r=new SemVer("0.0.0");if(e.test(r)){return r}r=new SemVer("0.0.0-0");if(e.test(r)){return r}r=null;for(var n=0;n<e.set.length;++n){var i=e.set[n];i.forEach(function(e){var t=new SemVer(e.semver.version);switch(e.operator){case">":if(t.prerelease.length===0){t.patch++}else{t.prerelease.push(0)}t.raw=t.format();case"":case">=":if(!r||gt(r,t)){r=t}break;case"<":case"<=":break;default:throw new Error("Unexpected operation: "+e.operator)}})}if(r&&e.test(r)){return r}return null}t.validRange=validRange;function validRange(e,t){try{return new Range(e,t).range||"*"}catch(e){return null}}t.ltr=ltr;function ltr(e,t,r){return outside(e,t,"<",r)}t.gtr=gtr;function gtr(e,t,r){return outside(e,t,">",r)}t.outside=outside;function outside(e,t,r,n){e=new SemVer(e,n);t=new Range(t,n);var i,o,s,a,c;switch(r){case">":i=gt;o=lte;s=lt;a=">";c=">=";break;case"<":i=lt;o=gte;s=gt;a="<";c="<=";break;default:throw new TypeError('Must provide a hilo val of "<" or ">"')}if(satisfies(e,t,n)){return false}for(var u=0;u<t.set.length;++u){var l=t.set[u];var f=null;var h=null;l.forEach(function(e){if(e.semver===v){e=new Comparator(">=0.0.0")}f=f||e;h=h||e;if(i(e.semver,f.semver,n)){f=e}else if(s(e.semver,h.semver,n)){h=e}});if(f.operator===a||f.operator===c){return false}if((!h.operator||h.operator===a)&&o(e,h.semver)){return false}else if(h.operator===c&&s(e,h.semver)){return false}}return true}t.prerelease=prerelease;function prerelease(e,t){var r=parse(e,t);return r&&r.prerelease.length?r.prerelease:null}t.intersects=intersects;function intersects(e,t,r){e=new Range(e,r);t=new Range(t,r);return e.intersects(t)}t.coerce=coerce;function coerce(e,t){if(e instanceof SemVer){return e}if(typeof e==="number"){e=String(e)}if(typeof e!=="string"){return null}t=t||{};var r=null;if(!t.rtl){r=e.match(s[c.COERCE])}else{var n;while((n=s[c.COERCERTL].exec(e))&&(!r||r.index+r[0].length!==e.length)){if(!r||n.index+n[0].length!==r.index+r[0].length){r=n}s[c.COERCERTL].lastIndex=n.index+n[1].length+n[2].length}s[c.COERCERTL].lastIndex=-1}if(r===null){return null}return parse(r[2]+"."+(r[3]||"0")+"."+(r[4]||"0"),t)}},281:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};Object.defineProperty(t,"__esModule",{value:true});const i=r(297);function create(e,t){return n(this,void 0,void 0,function*(){return yield i.DefaultGlobber.create(e,t)})}t.create=create},297:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__asyncValues||function(e){if(!Symbol.asyncIterator)throw new TypeError("Symbol.asyncIterator is not defined.");var t=e[Symbol.asyncIterator],r;return t?t.call(e):(e=typeof __values==="function"?__values(e):e[Symbol.iterator](),r={},verb("next"),verb("throw"),verb("return"),r[Symbol.asyncIterator]=function(){return this},r);function verb(t){r[t]=e[t]&&function(r){return new Promise(function(n,i){r=e[t](r),settle(n,i,r.done,r.value)})}}function settle(e,t,r,n){Promise.resolve(n).then(function(t){e({value:t,done:r})},t)}};var o=this&&this.__await||function(e){return this instanceof o?(this.v=e,this):new o(e)};var s=this&&this.__asyncGenerator||function(e,t,r){if(!Symbol.asyncIterator)throw new TypeError("Symbol.asyncIterator is not defined.");var n=r.apply(e,t||[]),i,s=[];return i={},verb("next"),verb("throw"),verb("return"),i[Symbol.asyncIterator]=function(){return this},i;function verb(e){if(n[e])i[e]=function(t){return new Promise(function(r,n){s.push([e,t,r,n])>1||resume(e,t)})}}function resume(e,t){try{step(n[e](t))}catch(e){settle(s[0][3],e)}}function step(e){e.value instanceof o?Promise.resolve(e.value.v).then(fulfill,reject):settle(s[0][2],e)}function fulfill(e){resume("next",e)}function reject(e){resume("throw",e)}function settle(e,t){if(e(t),s.shift(),s.length)resume(s[0][0],s[0][1])}};Object.defineProperty(t,"__esModule",{value:true});const a=r(470);const c=r(747);const u=r(601);const l=r(622);const f=r(597);const h=r(327);const p=r(923);const d=r(728);const v=process.platform==="win32";class DefaultGlobber{constructor(e){this.patterns=[];this.searchPaths=[];this.options=u.getOptions(e)}getSearchPaths(){return this.searchPaths.slice()}glob(){var e,t;return n(this,void 0,void 0,function*(){const r=[];try{for(var n=i(this.globGenerator()),o;o=yield n.next(),!o.done;){const e=o.value;r.push(e)}}catch(t){e={error:t}}finally{try{if(o&&!o.done&&(t=n.return))yield t.call(n)}finally{if(e)throw e.error}}return r})}globGenerator(){return s(this,arguments,function*globGenerator_1(){const e=u.getOptions(this.options);const t=[];for(const r of this.patterns){t.push(r);if(e.implicitDescendants&&(r.trailingSeparator||r.segments[r.segments.length-1]!=="**")){t.push(new p.Pattern(r.negate,r.segments.concat("**")))}}const r=[];for(const e of f.getSearchPaths(t)){a.debug(`Search path '${e}'`);try{yield o(c.promises.lstat(e))}catch(e){if(e.code==="ENOENT"){continue}throw e}r.unshift(new d.SearchState(e,1))}const n=[];while(r.length){const i=r.pop();const s=f.match(t,i.path);const a=!!s||f.partialMatch(t,i.path);if(!s&&!a){continue}const u=yield o(DefaultGlobber.stat(i,e,n));if(!u){continue}if(u.isDirectory()){if(s&h.MatchKind.Directory){yield yield o(i.path)}else if(!a){continue}const e=i.level+1;const t=(yield o(c.promises.readdir(i.path))).map(t=>new d.SearchState(l.join(i.path,t),e));r.push(...t.reverse())}else if(s&h.MatchKind.File){yield yield o(i.path)}}})}static create(e,t){return n(this,void 0,void 0,function*(){const r=new DefaultGlobber(t);if(v){e=e.replace(/\r\n/g,"\n");e=e.replace(/\r/g,"\n")}const n=e.split("\n").map(e=>e.trim());for(const e of n){if(!e||e.startsWith("#")){continue}else{r.patterns.push(new p.Pattern(e))}}r.searchPaths.push(...f.getSearchPaths(r.patterns));return r})}static stat(e,t,r){return n(this,void 0,void 0,function*(){let n;if(t.followSymbolicLinks){try{n=yield c.promises.stat(e.path)}catch(r){if(r.code==="ENOENT"){if(t.omitBrokenSymbolicLinks){a.debug(`Broken symlink '${e.path}'`);return undefined}throw new Error(`No information found for the path '${e.path}'. This may indicate a broken symbolic link.`)}throw r}}else{n=yield c.promises.lstat(e.path)}if(n.isDirectory()&&t.followSymbolicLinks){const t=yield c.promises.realpath(e.path);while(r.length>=e.level){r.pop()}if(r.some(e=>e===t)){a.debug(`Symlink cycle detected for path '${e.path}' and realpath '${t}'`);return undefined}r.push(t)}return n})}}t.DefaultGlobber=DefaultGlobber},306:function(e,t,r){var n=r(896);var i=r(621);e.exports=expandTop;var o="\0SLASH"+Math.random()+"\0";var s="\0OPEN"+Math.random()+"\0";var a="\0CLOSE"+Math.random()+"\0";var c="\0COMMA"+Math.random()+"\0";var u="\0PERIOD"+Math.random()+"\0";function numeric(e){return parseInt(e,10)==e?parseInt(e,10):e.charCodeAt(0)}function escapeBraces(e){return e.split("\\\\").join(o).split("\\{").join(s).split("\\}").join(a).split("\\,").join(c).split("\\.").join(u)}function unescapeBraces(e){return e.split(o).join("\\").split(s).join("{").split(a).join("}").split(c).join(",").split(u).join(".")}function parseCommaParts(e){if(!e)return[""];var t=[];var r=i("{","}",e);if(!r)return e.split(",");var n=r.pre;var o=r.body;var s=r.post;var a=n.split(",");a[a.length-1]+="{"+o+"}";var c=parseCommaParts(s);if(s.length){a[a.length-1]+=c.shift();a.push.apply(a,c)}t.push.apply(t,a);return t}function expandTop(e){if(!e)return[];if(e.substr(0,2)==="{}"){e="\\{\\}"+e.substr(2)}return expand(escapeBraces(e),true).map(unescapeBraces)}function identity(e){return e}function embrace(e){return"{"+e+"}"}function isPadded(e){return/^-?0\d/.test(e)}function lte(e,t){return e<=t}function gte(e,t){return e>=t}function expand(e,t){var r=[];var o=i("{","}",e);if(!o||/\$$/.test(o.pre))return[e];var s=/^-?\d+\.\.-?\d+(?:\.\.-?\d+)?$/.test(o.body);var c=/^[a-zA-Z]\.\.[a-zA-Z](?:\.\.-?\d+)?$/.test(o.body);var u=s||c;var l=o.body.indexOf(",")>=0;if(!u&&!l){if(o.post.match(/,.*\}/)){e=o.pre+"{"+o.body+a+o.post;return expand(e)}return[e]}var f;if(u){f=o.body.split(/\.\./)}else{f=parseCommaParts(o.body);if(f.length===1){f=expand(f[0],false).map(embrace);if(f.length===1){var h=o.post.length?expand(o.post,false):[""];return h.map(function(e){return o.pre+f[0]+e})}}}var p=o.pre;var h=o.post.length?expand(o.post,false):[""];var d;if(u){var v=numeric(f[0]);var y=numeric(f[1]);var g=Math.max(f[0].length,f[1].length);var m=f.length==3?Math.abs(numeric(f[2])):1;var E=lte;var S=y<v;if(S){m*=-1;E=gte}var R=f.some(isPadded);d=[];for(var w=v;E(w,y);w+=m){var C;if(c){C=String.fromCharCode(w);if(C==="\\")C=""}else{C=String(w);if(R){var b=g-C.length;if(b>0){var O=new Array(b+1).join("0");if(w<0)C="-"+O+C.slice(1);else C=O+C}}}d.push(C)}}else{d=n(f,function(e){return expand(e,false)})}for(var A=0;A<d.length;A++){for(var T=0;T<h.length;T++){var I=p+d[A]+h[T];if(!t||u||I)r.push(I)}}return r}},327:function(e,t){"use strict";Object.defineProperty(t,"__esModule",{value:true});var r;(function(e){e[e["None"]=0]="None";e[e["Directory"]=1]="Directory";e[e["File"]=2]="File";e[e["All"]=3]="All"})(r=t.MatchKind||(t.MatchKind={}))},357:function(e){e.exports=require("assert")},383:function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:true});const n=r(357);const i=r(622);const o=r(972);const s=process.platform==="win32";class Path{constructor(e){this.segments=[];if(typeof e==="string"){n(e,`Parameter 'itemPath' must not be empty`);e=o.safeTrimTrailingSeparator(e);if(!o.hasRoot(e)){this.segments=e.split(i.sep)}else{let t=e;let r=o.dirname(t);while(r!==t){const e=i.basename(t);this.segments.unshift(e);t=r;r=o.dirname(t)}this.segments.unshift(t)}}else{n(e.length>0,`Parameter 'itemPath' must not be an empty array`);for(let t=0;t<e.length;t++){let r=e[t];n(r,`Parameter 'itemPath' must not contain any empty segments`);r=o.normalizeSeparators(e[t]);if(t===0&&o.hasRoot(r)){r=o.safeTrimTrailingSeparator(r);n(r===o.dirname(r),`Parameter 'itemPath' root segment contains information for multiple segments`);this.segments.push(r)}else{n(!r.includes(i.sep),`Parameter 'itemPath' contains unexpected path separators`);this.segments.push(r)}}}}toString(){let e=this.segments[0];let t=e.endsWith(i.sep)||s&&/^[A-Z]:$/i.test(e);for(let r=1;r<this.segments.length;r++){if(t){t=false}else{e+=i.sep}e+=this.segments[r]}return e}}t.Path=Path},413:function(e,t,r){e.exports=r(141)},417:function(e){e.exports=require("crypto")},431:function(e,t,r){"use strict";var n=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const i=n(r(87));function issueCommand(e,t,r){const n=new Command(e,t,r);process.stdout.write(n.toString()+i.EOL)}t.issueCommand=issueCommand;function issue(e,t=""){issueCommand(e,{},t)}t.issue=issue;const o="::";class Command{constructor(e,t,r){if(!e){e="missing.command"}this.command=e;this.properties=t;this.message=r}toString(){let e=o+this.command;if(this.properties&&Object.keys(this.properties).length>0){e+=" ";let t=true;for(const r in this.properties){if(this.properties.hasOwnProperty(r)){const n=this.properties[r];if(n){if(t){t=false}else{e+=","}e+=`${r}=${escapeProperty(n)}`}}}}e+=`${o}${escapeData(this.message)}`;return e}}function toCommandValue(e){if(e===null||e===undefined){return""}else if(typeof e==="string"||e instanceof String){return e}return JSON.stringify(e)}t.toCommandValue=toCommandValue;function escapeData(e){return toCommandValue(e).replace(/%/g,"%25").replace(/\r/g,"%0D").replace(/\n/g,"%0A")}function escapeProperty(e){return toCommandValue(e).replace(/%/g,"%25").replace(/\r/g,"%0D").replace(/\n/g,"%0A").replace(/:/g,"%3A").replace(/,/g,"%2C")}},434:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=r(986);const s=i(r(1));const a=r(747);const c=i(r(622));const u=i(r(15));const l=r(931);function getTarPath(e,t){return n(this,void 0,void 0,function*(){const r=process.platform==="win32";if(r){const r=`${process.env["windir"]}\\System32\\tar.exe`;if(t!==l.CompressionMethod.Gzip){e.push("--force-local")}else if(a.existsSync(r)){return r}else if(yield u.isGnuTarInstalled()){e.push("--force-local")}}return yield s.which("tar",true)})}function execTar(e,t,r){return n(this,void 0,void 0,function*(){try{yield o.exec(`"${yield getTarPath(e,t)}"`,e,{cwd:r})}catch(e){throw new Error(`Tar failed with error: ${e===null||e===void 0?void 0:e.message}`)}})}function getWorkingDirectory(){var e;return(e=process.env["GITHUB_WORKSPACE"])!==null&&e!==void 0?e:process.cwd()}function extractTar(e,t){return n(this,void 0,void 0,function*(){const r=getWorkingDirectory();yield s.mkdirP(r);function getCompressionProgram(){switch(t){case l.CompressionMethod.Zstd:return["--use-compress-program","zstd -d --long=30"];case l.CompressionMethod.ZstdWithoutLong:return["--use-compress-program","zstd -d"];default:return["-z"]}}const n=[...getCompressionProgram(),"-xf",e.replace(new RegExp(`\\${c.sep}`,"g"),"/"),"-P","-C",r.replace(new RegExp(`\\${c.sep}`,"g"),"/")];yield execTar(n,t)})}t.extractTar=extractTar;function createTar(e,t,r){return n(this,void 0,void 0,function*(){const n="manifest.txt";const i=u.getCacheFileName(r);a.writeFileSync(c.join(e,n),t.join("\n"));const o=getWorkingDirectory();function getCompressionProgram(){switch(r){case l.CompressionMethod.Zstd:return["--use-compress-program","zstd -T0 --long=30"];case l.CompressionMethod.ZstdWithoutLong:return["--use-compress-program","zstd -T0"];default:return["-z"]}}const s=[...getCompressionProgram(),"-cf",i.replace(new RegExp(`\\${c.sep}`,"g"),"/"),"-P","-C",o.replace(new RegExp(`\\${c.sep}`,"g"),"/"),"--files-from",n];yield execTar(s,r,e)})}t.createTar=createTar},470:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=r(431);const s=i(r(87));const a=i(r(622));var c;(function(e){e[e["Success"]=0]="Success";e[e["Failure"]=1]="Failure"})(c=t.ExitCode||(t.ExitCode={}));function exportVariable(e,t){const r=o.toCommandValue(t);process.env[e]=r;o.issueCommand("set-env",{name:e},r)}t.exportVariable=exportVariable;function setSecret(e){o.issueCommand("add-mask",{},e)}t.setSecret=setSecret;function addPath(e){o.issueCommand("add-path",{},e);process.env["PATH"]=`${e}${a.delimiter}${process.env["PATH"]}`}t.addPath=addPath;function getInput(e,t){const r=process.env[`INPUT_${e.replace(/ /g,"_").toUpperCase()}`]||"";if(t&&t.required&&!r){throw new Error(`Input required and not supplied: ${e}`)}return r.trim()}t.getInput=getInput;function setOutput(e,t){o.issueCommand("set-output",{name:e},t)}t.setOutput=setOutput;function setCommandEcho(e){o.issue("echo",e?"on":"off")}t.setCommandEcho=setCommandEcho;function setFailed(e){process.exitCode=c.Failure;error(e)}t.setFailed=setFailed;function isDebug(){return process.env["RUNNER_DEBUG"]==="1"}t.isDebug=isDebug;function debug(e){o.issueCommand("debug",{},e)}t.debug=debug;function error(e){o.issue("error",e instanceof Error?e.toString():e)}t.error=error;function warning(e){o.issue("warning",e instanceof Error?e.toString():e)}t.warning=warning;function info(e){process.stdout.write(e+s.EOL)}t.info=info;function startGroup(e){o.issue("group",e)}t.startGroup=startGroup;function endGroup(){o.issue("endgroup")}t.endGroup=endGroup;function group(e,t){return n(this,void 0,void 0,function*(){startGroup(e);let r;try{r=yield t()}finally{endGroup()}return r})}t.group=group;function saveState(e,t){o.issueCommand("save-state",{name:e},t)}t.saveState=saveState;function getState(e){return process.env[`STATE_${e}`]||""}t.getState=getState},539:function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:true});const n=r(835);const i=r(605);const o=r(211);const s=r(950);let a;var c;(function(e){e[e["OK"]=200]="OK";e[e["MultipleChoices"]=300]="MultipleChoices";e[e["MovedPermanently"]=301]="MovedPermanently";e[e["ResourceMoved"]=302]="ResourceMoved";e[e["SeeOther"]=303]="SeeOther";e[e["NotModified"]=304]="NotModified";e[e["UseProxy"]=305]="UseProxy";e[e["SwitchProxy"]=306]="SwitchProxy";e[e["TemporaryRedirect"]=307]="TemporaryRedirect";e[e["PermanentRedirect"]=308]="PermanentRedirect";e[e["BadRequest"]=400]="BadRequest";e[e["Unauthorized"]=401]="Unauthorized";e[e["PaymentRequired"]=402]="PaymentRequired";e[e["Forbidden"]=403]="Forbidden";e[e["NotFound"]=404]="NotFound";e[e["MethodNotAllowed"]=405]="MethodNotAllowed";e[e["NotAcceptable"]=406]="NotAcceptable";e[e["ProxyAuthenticationRequired"]=407]="ProxyAuthenticationRequired";e[e["RequestTimeout"]=408]="RequestTimeout";e[e["Conflict"]=409]="Conflict";e[e["Gone"]=410]="Gone";e[e["TooManyRequests"]=429]="TooManyRequests";e[e["InternalServerError"]=500]="InternalServerError";e[e["NotImplemented"]=501]="NotImplemented";e[e["BadGateway"]=502]="BadGateway";e[e["ServiceUnavailable"]=503]="ServiceUnavailable";e[e["GatewayTimeout"]=504]="GatewayTimeout"})(c=t.HttpCodes||(t.HttpCodes={}));var u;(function(e){e["Accept"]="accept";e["ContentType"]="content-type"})(u=t.Headers||(t.Headers={}));var l;(function(e){e["ApplicationJson"]="application/json"})(l=t.MediaTypes||(t.MediaTypes={}));function getProxyUrl(e){let t=s.getProxyUrl(n.parse(e));return t?t.href:""}t.getProxyUrl=getProxyUrl;const f=[c.MovedPermanently,c.ResourceMoved,c.SeeOther,c.TemporaryRedirect,c.PermanentRedirect];const h=[c.BadGateway,c.ServiceUnavailable,c.GatewayTimeout];const p=["OPTIONS","GET","DELETE","HEAD"];const d=10;const v=5;class HttpClientResponse{constructor(e){this.message=e}readBody(){return new Promise(async(e,t)=>{let r=Buffer.alloc(0);this.message.on("data",e=>{r=Buffer.concat([r,e])});this.message.on("end",()=>{e(r.toString())})})}}t.HttpClientResponse=HttpClientResponse;function isHttps(e){let t=n.parse(e);return t.protocol==="https:"}t.isHttps=isHttps;class HttpClient{constructor(e,t,r){this._ignoreSslError=false;this._allowRedirects=true;this._allowRedirectDowngrade=false;this._maxRedirects=50;this._allowRetries=false;this._maxRetries=1;this._keepAlive=false;this._disposed=false;this.userAgent=e;this.handlers=t||[];this.requestOptions=r;if(r){if(r.ignoreSslError!=null){this._ignoreSslError=r.ignoreSslError}this._socketTimeout=r.socketTimeout;if(r.allowRedirects!=null){this._allowRedirects=r.allowRedirects}if(r.allowRedirectDowngrade!=null){this._allowRedirectDowngrade=r.allowRedirectDowngrade}if(r.maxRedirects!=null){this._maxRedirects=Math.max(r.maxRedirects,0)}if(r.keepAlive!=null){this._keepAlive=r.keepAlive}if(r.allowRetries!=null){this._allowRetries=r.allowRetries}if(r.maxRetries!=null){this._maxRetries=r.maxRetries}}}options(e,t){return this.request("OPTIONS",e,null,t||{})}get(e,t){return this.request("GET",e,null,t||{})}del(e,t){return this.request("DELETE",e,null,t||{})}post(e,t,r){return this.request("POST",e,t,r||{})}patch(e,t,r){return this.request("PATCH",e,t,r||{})}put(e,t,r){return this.request("PUT",e,t,r||{})}head(e,t){return this.request("HEAD",e,null,t||{})}sendStream(e,t,r,n){return this.request(e,t,r,n)}async getJson(e,t={}){t[u.Accept]=this._getExistingOrDefaultHeader(t,u.Accept,l.ApplicationJson);let r=await this.get(e,t);return this._processResponse(r,this.requestOptions)}async postJson(e,t,r={}){let n=JSON.stringify(t,null,2);r[u.Accept]=this._getExistingOrDefaultHeader(r,u.Accept,l.ApplicationJson);r[u.ContentType]=this._getExistingOrDefaultHeader(r,u.ContentType,l.ApplicationJson);let i=await this.post(e,n,r);return this._processResponse(i,this.requestOptions)}async putJson(e,t,r={}){let n=JSON.stringify(t,null,2);r[u.Accept]=this._getExistingOrDefaultHeader(r,u.Accept,l.ApplicationJson);r[u.ContentType]=this._getExistingOrDefaultHeader(r,u.ContentType,l.ApplicationJson);let i=await this.put(e,n,r);return this._processResponse(i,this.requestOptions)}async patchJson(e,t,r={}){let n=JSON.stringify(t,null,2);r[u.Accept]=this._getExistingOrDefaultHeader(r,u.Accept,l.ApplicationJson);r[u.ContentType]=this._getExistingOrDefaultHeader(r,u.ContentType,l.ApplicationJson);let i=await this.patch(e,n,r);return this._processResponse(i,this.requestOptions)}async request(e,t,r,i){if(this._disposed){throw new Error("Client has already been disposed.")}let o=n.parse(t);let s=this._prepareRequest(e,o,i);let a=this._allowRetries&&p.indexOf(e)!=-1?this._maxRetries+1:1;let u=0;let l;while(u<a){l=await this.requestRaw(s,r);if(l&&l.message&&l.message.statusCode===c.Unauthorized){let e;for(let t=0;t<this.handlers.length;t++){if(this.handlers[t].canHandleAuthentication(l)){e=this.handlers[t];break}}if(e){return e.handleAuthentication(this,s,r)}else{return l}}let t=this._maxRedirects;while(f.indexOf(l.message.statusCode)!=-1&&this._allowRedirects&&t>0){const a=l.message.headers["location"];if(!a){break}let c=n.parse(a);if(o.protocol=="https:"&&o.protocol!=c.protocol&&!this._allowRedirectDowngrade){throw new Error("Redirect from HTTPS to HTTP protocol. This downgrade is not allowed for security reasons. If you want to allow this behavior, set the allowRedirectDowngrade option to true.")}await l.readBody();if(c.hostname!==o.hostname){for(let e in i){if(e.toLowerCase()==="authorization"){delete i[e]}}}s=this._prepareRequest(e,c,i);l=await this.requestRaw(s,r);t--}if(h.indexOf(l.message.statusCode)==-1){return l}u+=1;if(u<a){await l.readBody();await this._performExponentialBackoff(u)}}return l}dispose(){if(this._agent){this._agent.destroy()}this._disposed=true}requestRaw(e,t){return new Promise((r,n)=>{let i=function(e,t){if(e){n(e)}r(t)};this.requestRawWithCallback(e,t,i)})}requestRawWithCallback(e,t,r){let n;if(typeof t==="string"){e.options.headers["Content-Length"]=Buffer.byteLength(t,"utf8")}let i=false;let o=(e,t)=>{if(!i){i=true;r(e,t)}};let s=e.httpModule.request(e.options,e=>{let t=new HttpClientResponse(e);o(null,t)});s.on("socket",e=>{n=e});s.setTimeout(this._socketTimeout||3*6e4,()=>{if(n){n.end()}o(new Error("Request timeout: "+e.options.path),null)});s.on("error",function(e){o(e,null)});if(t&&typeof t==="string"){s.write(t,"utf8")}if(t&&typeof t!=="string"){t.on("close",function(){s.end()});t.pipe(s)}else{s.end()}}getAgent(e){let t=n.parse(e);return this._getAgent(t)}_prepareRequest(e,t,r){const n={};n.parsedUrl=t;const s=n.parsedUrl.protocol==="https:";n.httpModule=s?o:i;const a=s?443:80;n.options={};n.options.host=n.parsedUrl.hostname;n.options.port=n.parsedUrl.port?parseInt(n.parsedUrl.port):a;n.options.path=(n.parsedUrl.pathname||"")+(n.parsedUrl.search||"");n.options.method=e;n.options.headers=this._mergeHeaders(r);if(this.userAgent!=null){n.options.headers["user-agent"]=this.userAgent}n.options.agent=this._getAgent(n.parsedUrl);if(this.handlers){this.handlers.forEach(e=>{e.prepareRequest(n.options)})}return n}_mergeHeaders(e){const t=e=>Object.keys(e).reduce((t,r)=>(t[r.toLowerCase()]=e[r],t),{});if(this.requestOptions&&this.requestOptions.headers){return Object.assign({},t(this.requestOptions.headers),t(e))}return t(e||{})}_getExistingOrDefaultHeader(e,t,r){const n=e=>Object.keys(e).reduce((t,r)=>(t[r.toLowerCase()]=e[r],t),{});let i;if(this.requestOptions&&this.requestOptions.headers){i=n(this.requestOptions.headers)[t]}return e[t]||i||r}_getAgent(e){let t;let n=s.getProxyUrl(e);let c=n&&n.hostname;if(this._keepAlive&&c){t=this._proxyAgent}if(this._keepAlive&&!c){t=this._agent}if(!!t){return t}const u=e.protocol==="https:";let l=100;if(!!this.requestOptions){l=this.requestOptions.maxSockets||i.globalAgent.maxSockets}if(c){if(!a){a=r(413)}const e={maxSockets:l,keepAlive:this._keepAlive,proxy:{proxyAuth:n.auth,host:n.hostname,port:n.port}};let i;const o=n.protocol==="https:";if(u){i=o?a.httpsOverHttps:a.httpsOverHttp}else{i=o?a.httpOverHttps:a.httpOverHttp}t=i(e);this._proxyAgent=t}if(this._keepAlive&&!t){const e={keepAlive:this._keepAlive,maxSockets:l};t=u?new o.Agent(e):new i.Agent(e);this._agent=t}if(!t){t=u?o.globalAgent:i.globalAgent}if(u&&this._ignoreSslError){t.options=Object.assign(t.options||{},{rejectUnauthorized:false})}return t}_performExponentialBackoff(e){e=Math.min(d,e);const t=v*Math.pow(2,e);return new Promise(e=>setTimeout(()=>e(),t))}static dateTimeDeserializer(e,t){if(typeof t==="string"){let e=new Date(t);if(!isNaN(e.valueOf())){return e}}return t}async _processResponse(e,t){return new Promise(async(r,n)=>{const i=e.message.statusCode;const o={statusCode:i,result:null,headers:{}};if(i==c.NotFound){r(o)}let s;let a;try{a=await e.readBody();if(a&&a.length>0){if(t&&t.deserializeDates){s=JSON.parse(a,HttpClient.dateTimeDeserializer)}else{s=JSON.parse(a)}o.result=s}o.headers=e.message.headers}catch(e){}if(i>299){let e;if(s&&s.message){e=s.message}else if(a&&a.length>0){e=a}else{e="Failed request: ("+i+")"}let t=new Error(e);t["statusCode"]=i;if(o.result){t["result"]=o.result}n(t)}else{r(o)}})}}t.HttpClient=HttpClient},597:function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:true});const n=r(972);const i=r(327);const o=process.platform==="win32";function getSearchPaths(e){e=e.filter(e=>!e.negate);const t={};for(const r of e){const e=o?r.searchPath.toUpperCase():r.searchPath;t[e]="candidate"}const r=[];for(const i of e){const e=o?i.searchPath.toUpperCase():i.searchPath;if(t[e]==="included"){continue}let s=false;let a=e;let c=n.dirname(a);while(c!==a){if(t[c]){s=true;break}a=c;c=n.dirname(a)}if(!s){r.push(i.searchPath);t[e]="included"}}return r}t.getSearchPaths=getSearchPaths;function match(e,t){let r=i.MatchKind.None;for(const n of e){if(n.negate){r&=~n.match(t)}else{r|=n.match(t)}}return r}t.match=match;function partialMatch(e,t){return e.some(e=>!e.negate&&e.partialMatch(t))}t.partialMatch=partialMatch},601:function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:true});const n=r(470);function getOptions(e){const t={followSymbolicLinks:true,implicitDescendants:true,omitBrokenSymbolicLinks:true};if(e){if(typeof e.followSymbolicLinks==="boolean"){t.followSymbolicLinks=e.followSymbolicLinks;n.debug(`followSymbolicLinks '${t.followSymbolicLinks}'`)}if(typeof e.implicitDescendants==="boolean"){t.implicitDescendants=e.implicitDescendants;n.debug(`implicitDescendants '${t.implicitDescendants}'`)}if(typeof e.omitBrokenSymbolicLinks==="boolean"){t.omitBrokenSymbolicLinks=e.omitBrokenSymbolicLinks;n.debug(`omitBrokenSymbolicLinks '${t.omitBrokenSymbolicLinks}'`)}}return t}t.getOptions=getOptions},605:function(e){e.exports=require("http")},614:function(e){e.exports=require("events")},621:function(e){"use strict";e.exports=balanced;function balanced(e,t,r){if(e instanceof RegExp)e=maybeMatch(e,r);if(t instanceof RegExp)t=maybeMatch(t,r);var n=range(e,t,r);return n&&{start:n[0],end:n[1],pre:r.slice(0,n[0]),body:r.slice(n[0]+e.length,n[1]),post:r.slice(n[1]+t.length)}}function maybeMatch(e,t){var r=t.match(e);return r?r[0]:null}balanced.range=range;function range(e,t,r){var n,i,o,s,a;var c=r.indexOf(e);var u=r.indexOf(t,c+1);var l=c;if(c>=0&&u>0){n=[];o=r.length;while(l>=0&&!a){if(l==c){n.push(l);c=r.indexOf(e,l+1)}else if(n.length==1){a=[n.pop(),u]}else{i=n.pop();if(i<o){o=i;s=u}u=r.indexOf(t,l+1)}l=c<u&&c>=0?c:u}if(n.length){a=[o,s]}}return a}},622:function(e){e.exports=require("path")},631:function(e){e.exports=require("net")},662:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__asyncValues||function(e){if(!Symbol.asyncIterator)throw new TypeError("Symbol.asyncIterator is not defined.");var t=e[Symbol.asyncIterator],r;return t?t.call(e):(e=typeof __values==="function"?__values(e):e[Symbol.iterator](),r={},verb("next"),verb("throw"),verb("return"),r[Symbol.asyncIterator]=function(){return this},r);function verb(t){r[t]=e[t]&&function(r){return new Promise(function(n,i){r=e[t](r),settle(n,i,r.done,r.value)})}}function settle(e,t,r,n){Promise.resolve(n).then(function(t){e({value:t,done:r})},t)}};var o=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const s=o(r(417));const a=o(r(747));const c=o(r(622));const u=o(r(794));const l=o(r(669));const f=o(r(281));function hashFiles(e,t=["**"],r=false){var o,h;var p,d,v,y;return n(this,void 0,void 0,function*(){let n=false;const g=s.createHash("sha256");try{for(o=i(t);h=yield o.next(),!h.done;){const t=h.value;const o=`${e}/${t}`;const p=yield f.create(o,{followSymbolicLinks:r});try{for(var m=i(p.globGenerator()),E;E=yield m.next(),!E.done;){const t=E.value;if(!t.startsWith(`${e}${c.sep}`)){continue}if(a.statSync(t).isDirectory()){continue}const r=s.createHash("sha256");const i=l.promisify(u.pipeline);yield i(a.createReadStream(t),r);g.write(r.digest());if(!n){n=true}}}catch(e){v={error:e}}finally{try{if(E&&!E.done&&(y=m.return))yield y.call(m)}finally{if(v)throw v.error}}}}catch(e){p={error:e}}finally{try{if(h&&!h.done&&(d=o.return))yield d.call(o)}finally{if(p)throw p.error}}g.end();return n?g.digest("hex"):null})}t.hashFiles=hashFiles},669:function(e){e.exports=require("util")},672:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i;Object.defineProperty(t,"__esModule",{value:true});const o=r(357);const s=r(747);const a=r(622);i=s.promises,t.chmod=i.chmod,t.copyFile=i.copyFile,t.lstat=i.lstat,t.mkdir=i.mkdir,t.readdir=i.readdir,t.readlink=i.readlink,t.rename=i.rename,t.rmdir=i.rmdir,t.stat=i.stat,t.symlink=i.symlink,t.unlink=i.unlink;t.IS_WINDOWS=process.platform==="win32";function exists(e){return n(this,void 0,void 0,function*(){try{yield t.stat(e)}catch(e){if(e.code==="ENOENT"){return false}throw e}return true})}t.exists=exists;function isDirectory(e,r=false){return n(this,void 0,void 0,function*(){const n=r?yield t.stat(e):yield t.lstat(e);return n.isDirectory()})}t.isDirectory=isDirectory;function isRooted(e){e=normalizeSeparators(e);if(!e){throw new Error('isRooted() parameter "p" cannot be empty')}if(t.IS_WINDOWS){return e.startsWith("\\")||/^[A-Z]:/i.test(e)}return e.startsWith("/")}t.isRooted=isRooted;function mkdirP(e,r=1e3,i=1){return n(this,void 0,void 0,function*(){o.ok(e,"a path argument must be provided");e=a.resolve(e);if(i>=r)return t.mkdir(e);try{yield t.mkdir(e);return}catch(n){switch(n.code){case"ENOENT":{yield mkdirP(a.dirname(e),r,i+1);yield t.mkdir(e);return}default:{let r;try{r=yield t.stat(e)}catch(e){throw n}if(!r.isDirectory())throw n}}}})}t.mkdirP=mkdirP;function tryGetExecutablePath(e,r){return n(this,void 0,void 0,function*(){let n=undefined;try{n=yield t.stat(e)}catch(t){if(t.code!=="ENOENT"){console.log(`Unexpected error attempting to determine if executable file exists '${e}': ${t}`)}}if(n&&n.isFile()){if(t.IS_WINDOWS){const t=a.extname(e).toUpperCase();if(r.some(e=>e.toUpperCase()===t)){return e}}else{if(isUnixExecutable(n)){return e}}}const i=e;for(const o of r){e=i+o;n=undefined;try{n=yield t.stat(e)}catch(t){if(t.code!=="ENOENT"){console.log(`Unexpected error attempting to determine if executable file exists '${e}': ${t}`)}}if(n&&n.isFile()){if(t.IS_WINDOWS){try{const r=a.dirname(e);const n=a.basename(e).toUpperCase();for(const i of yield t.readdir(r)){if(n===i.toUpperCase()){e=a.join(r,i);break}}}catch(t){console.log(`Unexpected error attempting to determine the actual case of the file '${e}': ${t}`)}return e}else{if(isUnixExecutable(n)){return e}}}}return""})}t.tryGetExecutablePath=tryGetExecutablePath;function normalizeSeparators(e){e=e||"";if(t.IS_WINDOWS){e=e.replace(/\//g,"\\");return e.replace(/\\\\+/g,"\\")}return e.replace(/\/\/+/g,"/")}function isUnixExecutable(e){return(e.mode&1)>0||(e.mode&8)>0&&e.gid===process.getgid()||(e.mode&64)>0&&e.uid===process.getuid()}},692:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=i(r(470));const s=i(r(622));const a=i(r(15));const c=i(r(114));const u=r(434);class ValidationError extends Error{constructor(e){super(e);this.name="ValidationError";Object.setPrototypeOf(this,ValidationError.prototype)}}t.ValidationError=ValidationError;class ReserveCacheError extends Error{constructor(e){super(e);this.name="ReserveCacheError";Object.setPrototypeOf(this,ReserveCacheError.prototype)}}t.ReserveCacheError=ReserveCacheError;function checkPaths(e){if(!e||e.length===0){throw new ValidationError(`Path Validation Error: At least one directory or file path is required`)}}function checkKey(e){if(e.length>512){throw new ValidationError(`Key Validation Error: ${e} cannot be larger than 512 characters.`)}const t=/^[^,]*$/;if(!t.test(e)){throw new ValidationError(`Key Validation Error: ${e} cannot contain commas.`)}}function restoreCache(e,t,r){return n(this,void 0,void 0,function*(){checkPaths(e);r=r||[];const n=[t,...r];o.debug("Resolved Keys:");o.debug(JSON.stringify(n));if(n.length>10){throw new ValidationError(`Key Validation Error: Keys are limited to a maximum of 10.`)}for(const e of n){checkKey(e)}const i=yield a.getCompressionMethod();const l=yield c.getCacheEntry(n,e,{compressionMethod:i});if(!(l===null||l===void 0?void 0:l.archiveLocation)){return undefined}const f=s.join(yield a.createTempDirectory(),a.getCacheFileName(i));o.debug(`Archive Path: ${f}`);try{yield c.downloadCache(l.archiveLocation,f);const e=a.getArchiveFileSizeIsBytes(f);o.info(`Cache Size: ~${Math.round(e/(1024*1024))} MB (${e} B)`);yield u.extractTar(f,i)}finally{try{yield a.unlinkFile(f)}catch(e){o.debug(`Failed to delete archive: ${e}`)}}return l.cacheKey})}t.restoreCache=restoreCache;function saveCache(e,t,r){return n(this,void 0,void 0,function*(){checkPaths(e);checkKey(t);const n=yield a.getCompressionMethod();o.debug("Reserving Cache");const i=yield c.reserveCache(t,e,{compressionMethod:n});if(i===-1){throw new ReserveCacheError(`Unable to reserve cache with key ${t}, another job may be creating this cache.`)}o.debug(`Cache ID: ${i}`);const l=yield a.resolvePaths(e);o.debug("Cache Paths:");o.debug(`${JSON.stringify(l)}`);const f=yield a.createTempDirectory();const h=s.join(f,a.getCacheFileName(n));o.debug(`Archive Path: ${h}`);yield u.createTar(f,l,n);const p=5*1024*1024*1024;const d=a.getArchiveFileSizeIsBytes(h);o.debug(`File Size: ${d}`);if(d>p){throw new Error(`Cache size of ~${Math.round(d/(1024*1024))} MB (${d} B) is over the 5GB limit, not saving cache.`)}o.debug(`Saving Cache (ID: ${i})`);yield c.saveCache(i,h,r);return i})}t.saveCache=saveCache},722:function(e){var t=[];for(var r=0;r<256;++r){t[r]=(r+256).toString(16).substr(1)}function bytesToUuid(e,r){var n=r||0;var i=t;return[i[e[n++]],i[e[n++]],i[e[n++]],i[e[n++]],"-",i[e[n++]],i[e[n++]],"-",i[e[n++]],i[e[n++]],"-",i[e[n++]],i[e[n++]],"-",i[e[n++]],i[e[n++]],i[e[n++]],i[e[n++]],i[e[n++]],i[e[n++]]].join("")}e.exports=bytesToUuid},728:function(e,t){"use strict";Object.defineProperty(t,"__esModule",{value:true});class SearchState{constructor(e,t){this.path=e;this.level=t}}t.SearchState=SearchState},747:function(e){e.exports=require("fs")},794:function(e){e.exports=require("stream")},826:function(e,t,r){var n=r(139);var i=r(722);function v4(e,t,r){var o=t&&r||0;if(typeof e=="string"){t=e==="binary"?new Array(16):null;e=null}e=e||{};var s=e.random||(e.rng||n)();s[6]=s[6]&15|64;s[8]=s[8]&63|128;if(t){for(var a=0;a<16;++a){t[o+a]=s[a]}}return t||i(s)}e.exports=v4},835:function(e){e.exports=require("url")},878:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=i(r(948));const s=i(r(167));function run(){return n(this,void 0,void 0,function*(){yield o.cacheWrapperDist();yield s.cacheDependencies()})}t.run=run;run()},888:function(e,t,r){"use strict";var n=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const i=n(r(470));function inputOrNull(e){const t=i.getInput(e,{required:false});if(t.length===0){return null}return t}t.inputOrNull=inputOrNull;function inputArrayOrNull(e){const t=inputOrNull(e);if(!t)return null;return t.split("\n").map(e=>e.trim()).filter(e=>e!=="")}t.inputArrayOrNull=inputArrayOrNull},896:function(e){e.exports=function(e,r){var n=[];for(var i=0;i<e.length;i++){var o=r(e[i],i);if(t(o))n.push.apply(n,o);else n.push(o)}return n};var t=Array.isArray||function(e){return Object.prototype.toString.call(e)==="[object Array]"}},898:function(e,t,r){var n=r(86);var i=r(826);var o=i;o.v1=n;o.v4=i;e.exports=o},923:function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:true});const n=r(357);const i=r(87);const o=r(622);const s=r(972);const a=r(93);const c=r(327);const u=r(383);const l=process.platform==="win32";class Pattern{constructor(e,t){this.negate=false;let r;if(typeof e==="string"){r=e.trim()}else{t=t||[];n(t.length,`Parameter 'segments' must not empty`);const i=Pattern.getLiteral(t[0]);n(i&&s.hasAbsoluteRoot(i),`Parameter 'segments' first element must be a root path`);r=new u.Path(t).toString().trim();if(e){r=`!${r}`}}while(r.startsWith("!")){this.negate=!this.negate;r=r.substr(1).trim()}r=Pattern.fixupPattern(r);this.segments=new u.Path(r).segments;this.trailingSeparator=s.normalizeSeparators(r).endsWith(o.sep);r=s.safeTrimTrailingSeparator(r);let i=false;const c=this.segments.map(e=>Pattern.getLiteral(e)).filter(e=>!i&&!(i=e===""));this.searchPath=new u.Path(c).toString();this.rootRegExp=new RegExp(Pattern.regExpEscape(c[0]),l?"i":"");const f={dot:true,nobrace:true,nocase:l,nocomment:true,noext:true,nonegate:true};r=l?r.replace(/\\/g,"/"):r;this.minimatch=new a.Minimatch(r,f)}match(e){if(this.segments[this.segments.length-1]==="**"){e=s.normalizeSeparators(e);if(!e.endsWith(o.sep)){e=`${e}${o.sep}`}}else{e=s.safeTrimTrailingSeparator(e)}if(this.minimatch.match(e)){return this.trailingSeparator?c.MatchKind.Directory:c.MatchKind.All}return c.MatchKind.None}partialMatch(e){e=s.safeTrimTrailingSeparator(e);if(s.dirname(e)===e){return this.rootRegExp.test(e)}return this.minimatch.matchOne(e.split(l?/\\+/:/\/+/),this.minimatch.set[0],true)}static globEscape(e){return(l?e:e.replace(/\\/g,"\\\\")).replace(/(\[)(?=[^/]+\])/g,"[[]").replace(/\?/g,"[?]").replace(/\*/g,"[*]")}static fixupPattern(e){n(e,"pattern cannot be empty");const t=new u.Path(e).segments.map(e=>Pattern.getLiteral(e));n(t.every((e,t)=>(e!=="."||t===0)&&e!==".."),`Invalid pattern '${e}'. Relative pathing '.' and '..' is not allowed.`);n(!s.hasRoot(e)||t[0],`Invalid pattern '${e}'. Root segment must not contain globs.`);e=s.normalizeSeparators(e);if(e==="."||e.startsWith(`.${o.sep}`)){e=Pattern.globEscape(process.cwd())+e.substr(1)}else if(e==="~"||e.startsWith(`~${o.sep}`)){const t=i.homedir();n(t,"Unable to determine HOME directory");n(s.hasAbsoluteRoot(t),`Expected HOME directory to be a rooted path. Actual '${t}'`);e=Pattern.globEscape(t)+e.substr(1)}else if(l&&(e.match(/^[A-Z]:$/i)||e.match(/^[A-Z]:[^\\]/i))){let t=s.ensureAbsoluteRoot("C:\\dummy-root",e.substr(0,2));if(e.length>2&&!t.endsWith("\\")){t+="\\"}e=Pattern.globEscape(t)+e.substr(2)}else if(l&&(e==="\\"||e.match(/^\\[^\\]/))){let t=s.ensureAbsoluteRoot("C:\\dummy-root","\\");if(!t.endsWith("\\")){t+="\\"}e=Pattern.globEscape(t)+e.substr(1)}else{e=s.ensureAbsoluteRoot(Pattern.globEscape(process.cwd()),e)}return s.normalizeSeparators(e)}static getLiteral(e){let t="";for(let r=0;r<e.length;r++){const n=e[r];if(n==="\\"&&!l&&r+1<e.length){t+=e[++r];continue}else if(n==="*"||n==="?"){return""}else if(n==="["&&r+1<e.length){let n="";let i=-1;for(let t=r+1;t<e.length;t++){const r=e[t];if(r==="\\"&&!l&&t+1<e.length){n+=e[++t];continue}else if(r==="]"){i=t;break}else{n+=r}}if(i>=0){if(n.length>1){return""}if(n){t+=n;r=i;continue}}}t+=n}return t}static regExpEscape(e){return e.replace(/[[\\^$.|?*+()]/g,"\\$&")}}t.Pattern=Pattern},931:function(e,t){"use strict";Object.defineProperty(t,"__esModule",{value:true});var r;(function(e){e["Gzip"]="cache.tgz";e["Zstd"]="cache.tzst"})(r=t.CacheFilename||(t.CacheFilename={}));var n;(function(e){e["Gzip"]="gzip";e["ZstdWithoutLong"]="zstd-without-long";e["Zstd"]="zstd"})(n=t.CompressionMethod||(t.CompressionMethod={}));t.SocketTimeout=5e3},948:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=i(r(470));const s=i(r(692));const a=i(r(622));const c=i(r(747));const u=i(r(87));const l="WRAPPER_CACHE_KEY";const f="WRAPPER_CACHE_PATH";const h="WRAPPER_CACHE_RESULT";function restoreCachedWrapperDist(e){return n(this,void 0,void 0,function*(){if(e==null)return;const t=extractGradleWrapperSlugFrom(a.join(a.resolve(e),"gradle/wrapper/gradle-wrapper.properties"));if(!t)return;const r=`wrapper-${t}`;const n=a.join(u.homedir(),`.gradle/wrapper/dists/gradle-${t}`);o.saveState(l,r);o.saveState(f,n);const i=yield s.restoreCache([n],r);if(!i){o.info("Wrapper installation cache not found, expect a Gradle distribution download.");return}o.saveState(h,i);o.info(`Wrapper installation restored from cache key: ${i}`);return})}t.restoreCachedWrapperDist=restoreCachedWrapperDist;function cacheWrapperDist(){return n(this,void 0,void 0,function*(){const e=o.getState(l);const t=o.getState(f);const r=o.getState(h);if(!t||!c.existsSync(t)){o.debug("No wrapper installation to cache.");return}if(r&&e===r){o.info(`Wrapper installation cache hit occurred on the cache key ${e}, not saving cache.`);return}try{yield s.saveCache([t],e)}catch(e){if(e.name===s.ValidationError.name){throw e}else if(e.name===s.ReserveCacheError.name){o.info(e.message)}else{o.info(`[warning] ${e.message}`)}}return})}t.cacheWrapperDist=cacheWrapperDist;function extractGradleWrapperSlugFrom(e){const t=c.readFileSync(e,{encoding:"utf8"});const r=t.split("\n").find(e=>e.startsWith("distributionUrl"));if(!r)return null;return extractGradleWrapperSlugFromDistUri(r.substr(16).trim())}t.extractGradleWrapperSlugFrom=extractGradleWrapperSlugFrom;function extractGradleWrapperSlugFromDistUri(e){const t=/.*gradle-(.*-(bin|all))\.zip/;const r=e.match(t);return r?r[1]:null}t.extractGradleWrapperSlugFromDistUri=extractGradleWrapperSlugFromDistUri},950:function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:true});const n=r(835);function getProxyUrl(e){let t=e.protocol==="https:";let r;if(checkBypass(e)){return r}let i;if(t){i=process.env["https_proxy"]||process.env["HTTPS_PROXY"]}else{i=process.env["http_proxy"]||process.env["HTTP_PROXY"]}if(i){r=n.parse(i)}return r}t.getProxyUrl=getProxyUrl;function checkBypass(e){if(!e.hostname){return false}let t=process.env["no_proxy"]||process.env["NO_PROXY"]||"";if(!t){return false}let r;if(e.port){r=Number(e.port)}else if(e.protocol==="http:"){r=80}else if(e.protocol==="https:"){r=443}let n=[e.hostname.toUpperCase()];if(typeof r==="number"){n.push(`${n[0]}:${r}`)}for(let e of t.split(",").map(e=>e.trim().toUpperCase()).filter(e=>e)){if(n.some(t=>t===e)){return true}}return false}t.checkBypass=checkBypass},972:function(e,t,r){"use strict";Object.defineProperty(t,"__esModule",{value:true});const n=r(357);const i=r(622);const o=process.platform==="win32";function dirname(e){e=safeTrimTrailingSeparator(e);if(o&&/^\\\\[^\\]+(\\[^\\]+)?$/.test(e)){return e}let t=i.dirname(e);if(o&&/^\\\\[^\\]+\\[^\\]+\\$/.test(t)){t=safeTrimTrailingSeparator(t)}return t}t.dirname=dirname;function ensureAbsoluteRoot(e,t){n(e,`ensureAbsoluteRoot parameter 'root' must not be empty`);n(t,`ensureAbsoluteRoot parameter 'itemPath' must not be empty`);if(hasAbsoluteRoot(t)){return t}if(o){if(t.match(/^[A-Z]:[^\\/]|^[A-Z]:$/i)){let e=process.cwd();n(e.match(/^[A-Z]:\\/i),`Expected current directory to start with an absolute drive root. Actual '${e}'`);if(t[0].toUpperCase()===e[0].toUpperCase()){if(t.length===2){return`${t[0]}:\\${e.substr(3)}`}else{if(!e.endsWith("\\")){e+="\\"}return`${t[0]}:\\${e.substr(3)}${t.substr(2)}`}}else{return`${t[0]}:\\${t.substr(2)}`}}else if(normalizeSeparators(t).match(/^\\$|^\\[^\\]/)){const e=process.cwd();n(e.match(/^[A-Z]:\\/i),`Expected current directory to start with an absolute drive root. Actual '${e}'`);return`${e[0]}:\\${t.substr(1)}`}}n(hasAbsoluteRoot(e),`ensureAbsoluteRoot parameter 'root' must have an absolute root`);if(e.endsWith("/")||o&&e.endsWith("\\")){}else{e+=i.sep}return e+t}t.ensureAbsoluteRoot=ensureAbsoluteRoot;function hasAbsoluteRoot(e){n(e,`hasAbsoluteRoot parameter 'itemPath' must not be empty`);e=normalizeSeparators(e);if(o){return e.startsWith("\\\\")||/^[A-Z]:\\/i.test(e)}return e.startsWith("/")}t.hasAbsoluteRoot=hasAbsoluteRoot;function hasRoot(e){n(e,`isRooted parameter 'itemPath' must not be empty`);e=normalizeSeparators(e);if(o){return e.startsWith("\\")||/^[A-Z]:/i.test(e)}return e.startsWith("/")}t.hasRoot=hasRoot;function normalizeSeparators(e){e=e||"";if(o){e=e.replace(/\//g,"\\");const t=/^\\\\+[^\\]/.test(e);return(t?"\\":"")+e.replace(/\\\\+/g,"\\")}return e.replace(/\/\/+/g,"/")}t.normalizeSeparators=normalizeSeparators;function safeTrimTrailingSeparator(e){if(!e){return""}e=normalizeSeparators(e);if(!e.endsWith(i.sep)){return e}if(e===i.sep){return e}if(o&&/^[A-Z]:\\$/i.test(e)){return e}return e.substr(0,e.length-1)}t.safeTrimTrailingSeparator=safeTrimTrailingSeparator},986:function(e,t,r){"use strict";var n=this&&this.__awaiter||function(e,t,r,n){function adopt(e){return e instanceof r?e:new r(function(t){t(e)})}return new(r||(r=Promise))(function(r,i){function fulfilled(e){try{step(n.next(e))}catch(e){i(e)}}function rejected(e){try{step(n["throw"](e))}catch(e){i(e)}}function step(e){e.done?r(e.value):adopt(e.value).then(fulfilled,rejected)}step((n=n.apply(e,t||[])).next())})};var i=this&&this.__importStar||function(e){if(e&&e.__esModule)return e;var t={};if(e!=null)for(var r in e)if(Object.hasOwnProperty.call(e,r))t[r]=e[r];t["default"]=e;return t};Object.defineProperty(t,"__esModule",{value:true});const o=i(r(9));function exec(e,t,r){return n(this,void 0,void 0,function*(){const n=o.argStringToArray(e);if(n.length===0){throw new Error(`Parameter 'commandLine' cannot be null or empty.`)}const i=n[0];t=n.slice(1).concat(t||[]);const s=new o.ToolRunner(i,t,r);return s.exec()})}t.exec=exec}}); |